volume
MakieCore.volume Function
volume(volume_data)
volume(x, y, z, volume_data)
Plots a volume, with optional physical dimensions x, y, z
. Available algorithms are:
:iso
=> IsoValue:absorption
=> Absorption:mip
=> MaximumIntensityProjection:absorptionrgba
=> AbsorptionRGBA:additive
=> AdditiveRGBA:indexedabsorption
=> IndexedAbsorptionRGBA
Plot type
The plot type alias for the volume
function is Volume
.
Examples
using GLMakie
r = LinRange(-1, 1, 100)
cube = [(x.^2 + y.^2 + z.^2) for x = r, y = r, z = r]
contour(cube, alpha=0.5)
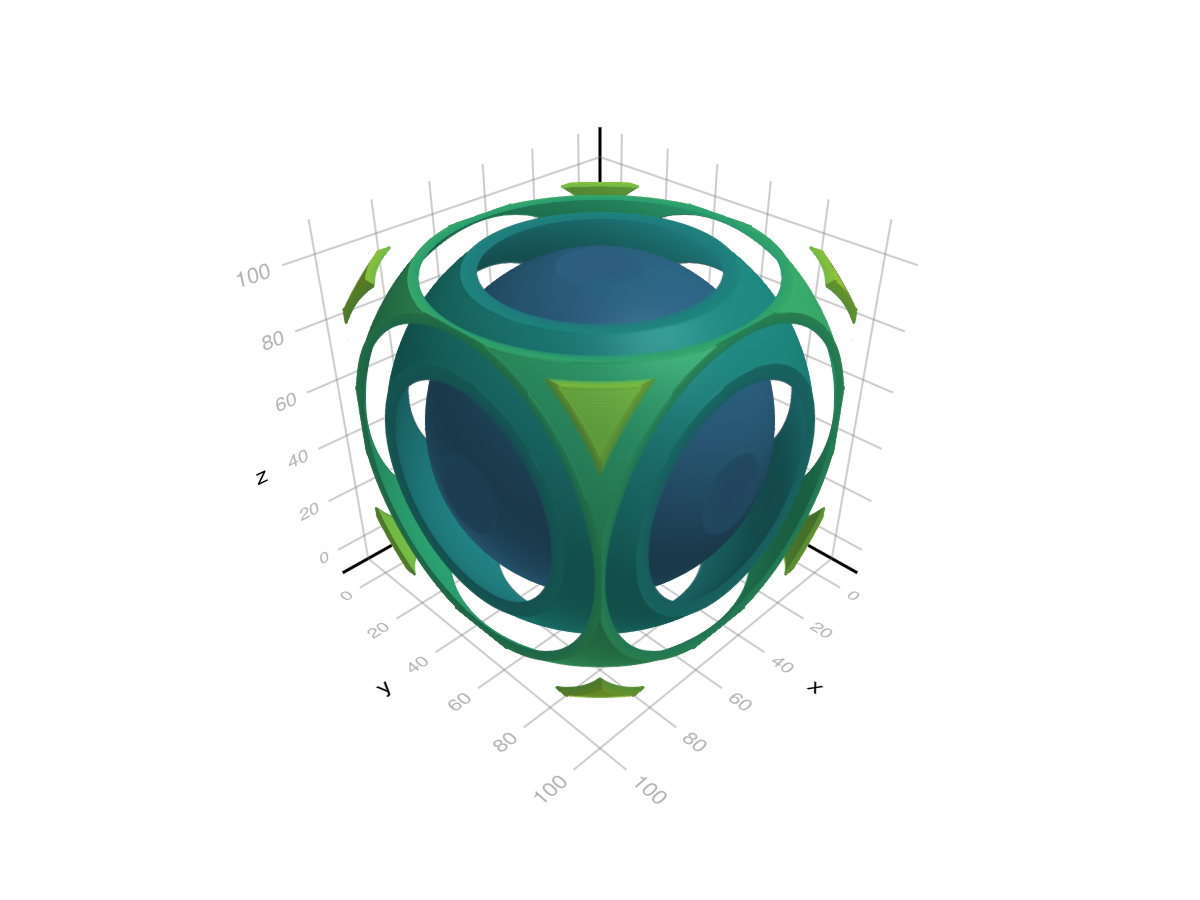
cube_with_holes = cube .* (cube .> 1.4)
volume(cube_with_holes, algorithm = :iso, isorange = 0.05, isovalue = 1.7)
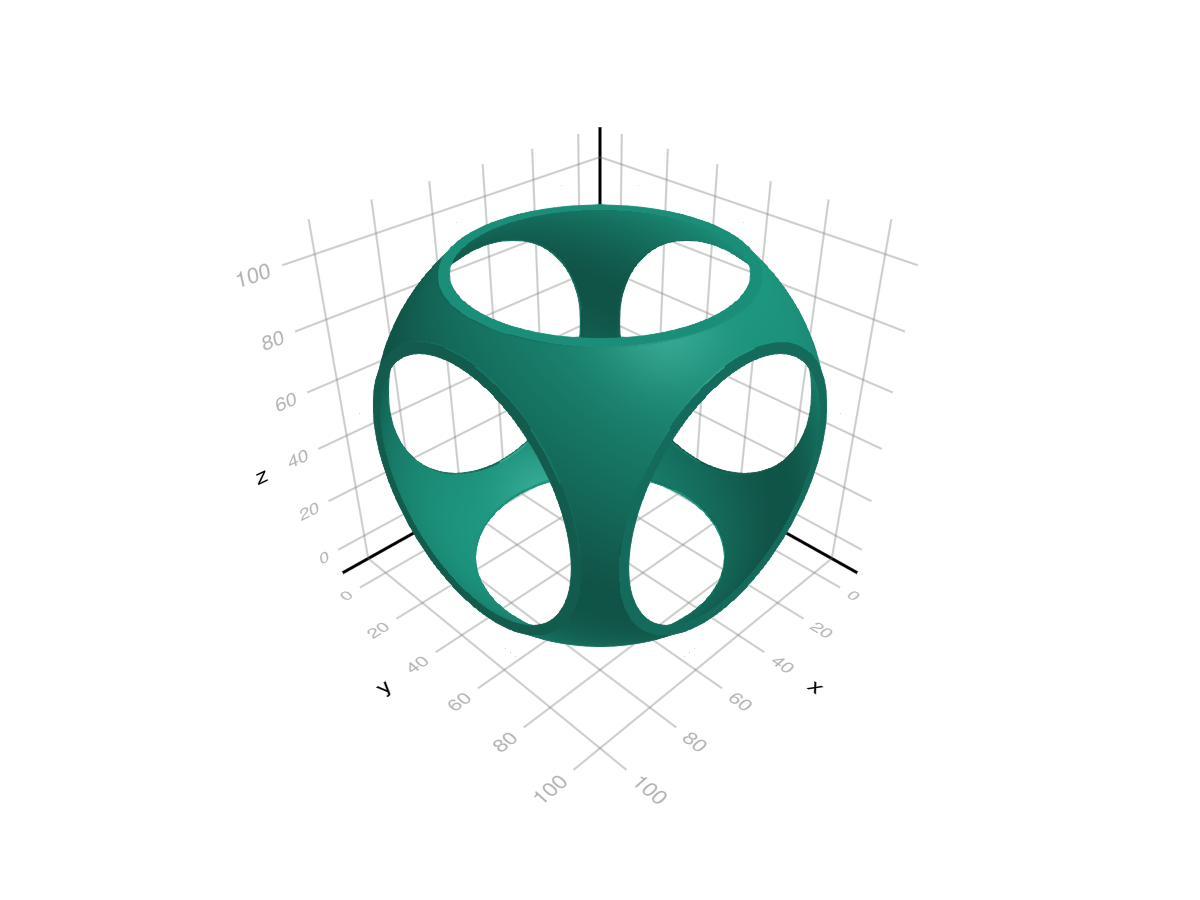
using GLMakie
using NIfTI
brain = niread(Makie.assetpath("brain.nii.gz")).raw
mini, maxi = extrema(brain)
normed = Float32.((brain .- mini) ./ (maxi - mini))
fig = Figure(size=(1000, 450))
# Make a colormap, with the first value being transparent
colormap = to_colormap(:plasma)
colormap[1] = RGBAf(0,0,0,0)
volume(fig[1, 1], normed, algorithm = :absorption, absorption=4f0, colormap=colormap, axis=(type=Axis3, title = "Absorption"))
volume(fig[1, 2], normed, algorithm = :mip, colormap=colormap, axis=(type=Axis3, title="Maximum Intensity Projection"))
fig
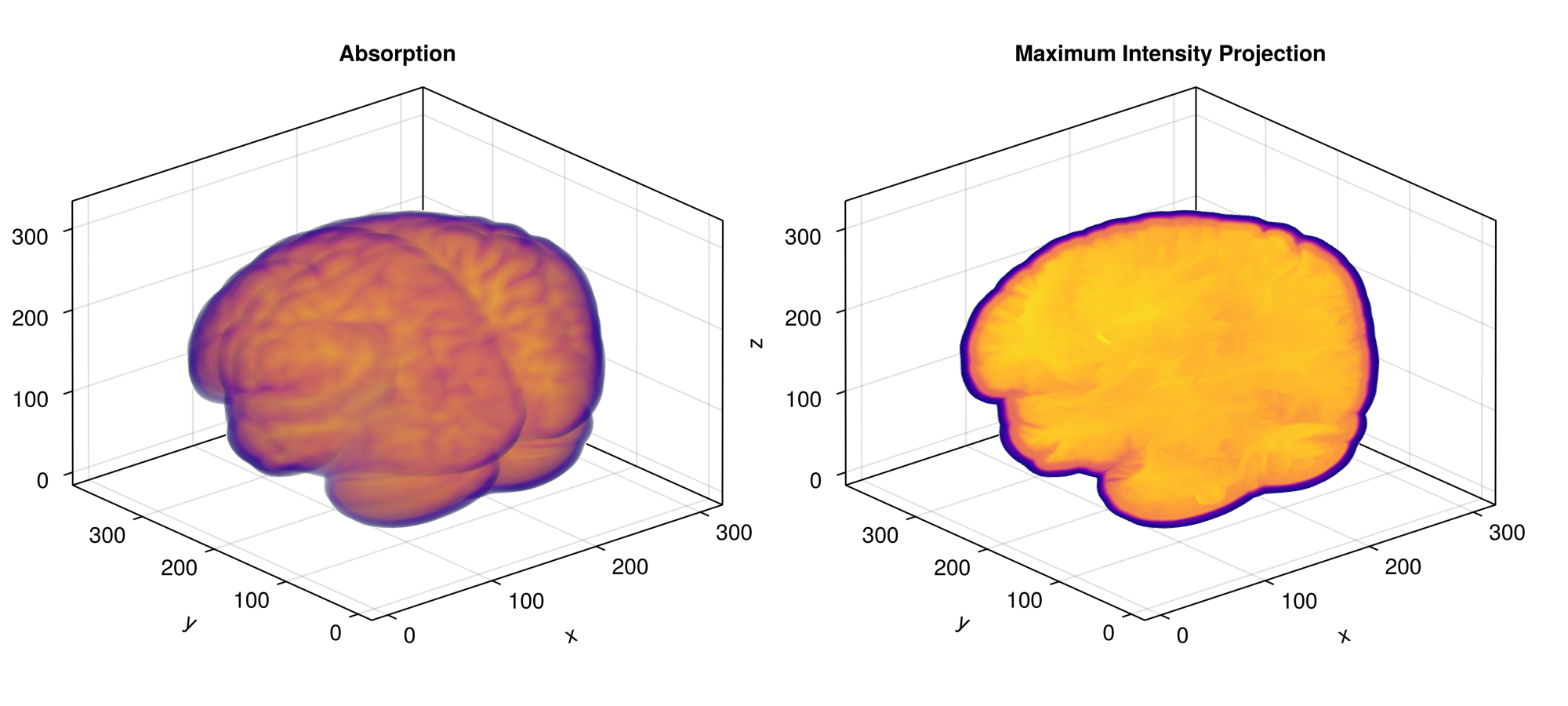
Attributes
absorption
Defaults to 1.0
Absorption multiplier for algorithm=:absorption. This changes how much light each voxel absorbs.
algorithm
Defaults to :mip
Sets the volume algorithm that is used.
alpha
Defaults to 1.0
The alpha value of the colormap or color attribute. Multiple alphas like in plot(alpha=0.2, color=(:red, 0.5)
, will get multiplied.
backlight
Defaults to 0.0
Sets a weight for secondary light calculation with inverted normals.
clip_planes
Defaults to automatic
Clip planes offer a way to do clipping in 3D space. You can set a Vector of up to 8 Plane3f
planes here, behind which plots will be clipped (i.e. become invisible). By default clip planes are inherited from the parent plot or scene. You can remove parent clip_planes
by passing Plane3f[]
.
colormap
Defaults to @inherit colormap :viridis
Sets the colormap that is sampled for numeric color
s. PlotUtils.cgrad(...)
, Makie.Reverse(any_colormap)
can be used as well, or any symbol from ColorBrewer or PlotUtils. To see all available color gradients, you can call Makie.available_gradients()
.
colorrange
Defaults to automatic
The values representing the start and end points of colormap
.
colorscale
Defaults to identity
The color transform function. Can be any function, but only works well together with Colorbar
for identity
, log
, log2
, log10
, sqrt
, logit
, Makie.pseudolog10
and Makie.Symlog10
.
depth_shift
Defaults to 0.0
adjusts the depth value of a plot after all other transformations, i.e. in clip space, where 0 <= depth <= 1
. This only applies to GLMakie and WGLMakie and can be used to adjust render order (like a tunable overdraw).
diffuse
Defaults to 1.0
Sets how strongly the red, green and blue channel react to diffuse (scattered) light.
enable_depth
Defaults to true
Enables depth write for Volume, so that volume correctly occludes other objects.
fxaa
Defaults to true
adjusts whether the plot is rendered with fxaa (anti-aliasing, GLMakie only).
highclip
Defaults to automatic
The color for any value above the colorrange.
inspectable
Defaults to true
sets whether this plot should be seen by DataInspector
.
inspector_clear
Defaults to automatic
Sets a callback function (inspector, plot) -> ...
for cleaning up custom indicators in DataInspector.
inspector_hover
Defaults to automatic
Sets a callback function (inspector, plot, index) -> ...
which replaces the default show_data
methods.
inspector_label
Defaults to automatic
Sets a callback function (plot, index, position) -> string
which replaces the default label generated by DataInspector.
interpolate
Defaults to true
Sets whether the volume data should be sampled with interpolation.
isorange
Defaults to 0.05
Sets the range of values picked up by the IsoValue algorithm.
isovalue
Defaults to 0.5
Sets the target value for the IsoValue algorithm.
lowclip
Defaults to automatic
The color for any value below the colorrange.
material
Defaults to nothing
RPRMakie only attribute to set complex RadeonProRender materials. Warning, how to set an RPR material may change and other backends will ignore this attribute
model
Defaults to automatic
Sets a model matrix for the plot. This overrides adjustments made with translate!
, rotate!
and scale!
.
nan_color
Defaults to :transparent
The color for NaN values.
overdraw
Defaults to false
Controls if the plot will draw over other plots. This specifically means ignoring depth checks in GL backends
shading
Defaults to automatic
Sets the lighting algorithm used. Options are NoShading
(no lighting), FastShading
(AmbientLight + PointLight) or MultiLightShading
(Multiple lights, GLMakie only). Note that this does not affect RPRMakie.
shininess
Defaults to 32.0
Sets how sharp the reflection is.
space
Defaults to :data
sets the transformation space for box encompassing the plot. See Makie.spaces()
for possible inputs.
specular
Defaults to 0.2
Sets how strongly the object reflects light in the red, green and blue channels.
ssao
Defaults to false
Adjusts whether the plot is rendered with ssao (screen space ambient occlusion). Note that this only makes sense in 3D plots and is only applicable with fxaa = true
.
transformation
Defaults to automatic
No docs available.
transparency
Defaults to false
Adjusts how the plot deals with transparency. In GLMakie transparency = true
results in using Order Independent Transparency.
visible
Defaults to true
Controls whether the plot will be rendered or not.