Colors
There are multiple ways to specify colors in Makie. Most plot objects allow passing an array as the color
attribute, where there must be as many color elements as there are visual elements (scatter markers, line segments, polygons, etc.). Alternatively, one can pass a single color element which is applied to all visual elements at once.
When passing an array of numbers or a single number, the values are converted to colors using the colormap
and colorrange
attributes. By default, the colorrange
spans the range of the color values, but it can be fixed manually as well. For example, this can be useful for picking categorical colors. The number 1
will pick the first and the number 2
the second color from the 10-color categorical map :tab10
, for example, if the colorrange
is set to (1, 10)
.
NaN
values are usually displayed with :transparent
color, so they are invisible. This can be changed with the attribute nan_color
.
If values exceed the colorrange
at the low or high end, by default the start or end color of the map is picked, unless the lowclip
and highclip
attributes are set to some other color.
Alpha or Opacity
You can use alpha
keyword in most Makie Plots.
Alternatively, one can make partially transparent colors or colormaps by passing a tuple (color, alpha)
to the color/colormap attribute.
Textures, Patterns and MatCaps
Some plot types (e.g. mesh, surface, ...) allow you to sample colors from an image. The sampling can happen based on texture coordinates (uv coordinates), pixel coordinates or normals.
The first case is used when an image Matrix
is passed directly as the color
attribute. Note that texture coordinates need to be available to get a well defined result.
The second case is used when a Makie.AbstractPattern
is passed as the color
. This is typically used for hatching. For example a hatching pattern with diagonal lines can be set with color = Pattern('/')
. More generally, you can define a line pattern with Makie.LinePattern()
or use an image as a pattern with Pattern(image)
.
The last case is used when an image is passed with the matcap
attribute. The image is then interpreted as going from (-1, 1) to (1, 1) so that normals can be mapped to it. The (0,0,1) direction of the normal is facing the camera/viewer.
using GLMakie
using GLMakie, FileIO
f = Figure()
mesh(f[1, 1], Rect2f(0,0,1,1), color = load(Makie.assetpath("cow.png")), shading = NoShading, axis=(title ="texture",))
mesh(f[2, 1], Rect2f(0,0,1,1), color = Pattern('/'), shading = NoShading, axis=(title ="Pattern",))
hidedecorations!.(f.content)
catmesh = FileIO.load(assetpath("cat.obj"))
texture = FileIO.load(assetpath("diffusemap.png"))
matcap = FileIO.load(Base.download("https://raw.githubusercontent.com/nidorx/matcaps/master/1024/E6BF3C_5A4719_977726_FCFC82.png"))
Label(f[1, 2][1, 1], "texture 3D", tellwidth = false)
a, p = mesh(f[1, 2][2, 1], catmesh, color = texture, axis = (show_axis = false, ))
Label(f[2, 2][1, 1], "matcap", tellwidth = false)
mesh(f[2, 2][2, 1], catmesh, matcap = matcap, shading = NoShading, axis = (show_axis = false, ))
f
┌ Error: obj file contains references to .mtl files, but none could be found. Expected: ["cat.mtl"] in /home/runner/work/Makie.jl/Makie.jl/assets.
└ @ MeshIO ~/.julia/packages/MeshIO/jBkmz/src/io/obj.jl:157
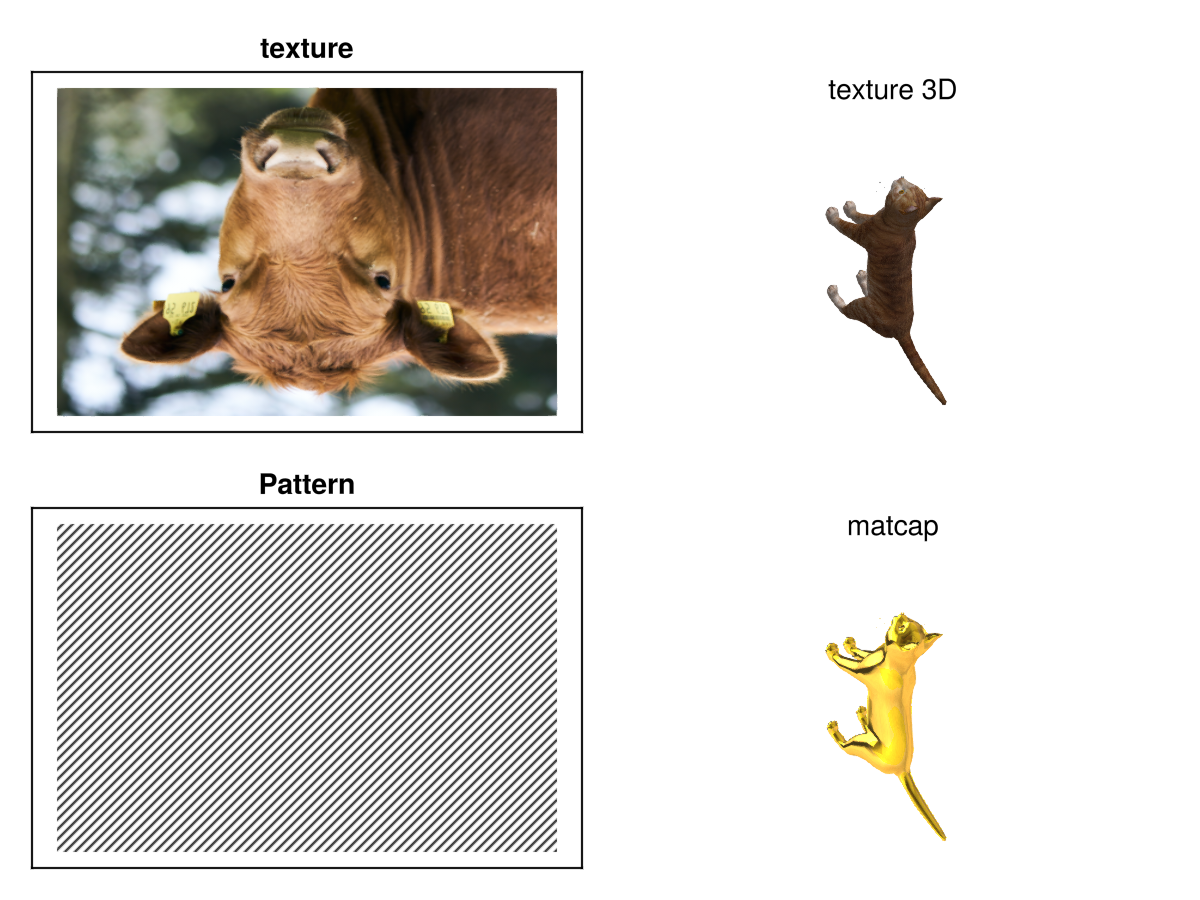
Cheat Sheet
Here's a little cheat sheet showing common color specifications:
using CairoMakie
using Makie.Colors
theme = Attributes(
Scatter = (; markersize = 40),
Text = (; align = (:center, :bottom), offset = (0, 30))
)
with_theme(theme) do
f = Figure(size = (800, 1200))
ax = Axis(f[1, 1], xautolimitmargin = (0.2, 0.2), yautolimitmargin = (0.1, 0.1))
hidedecorations!(ax)
hidespines!(ax)
scatter!(ax, 1, 1, color = :red)
text!(ax, 1, 1, text = ":red")
scatter!(ax, 2, 1, color = (:red, 0.5))
text!(ax, 2, 1, text = "(:red, 0.5)")
scatter!(ax, 3, 1, color = RGBf(0.5, 0.2, 0.8))
text!(ax, 3, 1, text = "RGBf(0.5, 0.2, 0.8)")
scatter!(ax, 4, 1, color = RGBAf(0.5, 0.2, 0.8, 0.5))
text!(ax, 4, 1, text = "RGBAf(0.5, 0.2, 0.8, 0.5)")
scatter!(ax, 1, 0, color = Colors.HSV(40, 30, 60))
text!(ax, 1, 0, text = "Colors.HSV(40, 30, 60)")
scatter!(ax, 2, 0, color = 1, colormap = :tab10, colorrange = (1, 10))
text!(ax, 2, 0, text = "color = 1\ncolormap = :tab10\ncolorrange = (1, 10)")
scatter!(ax, 3, 0, color = 2, colormap = :tab10, colorrange = (1, 10))
text!(ax, 3, 0, text = "color = 2\ncolormap = :tab10\ncolorrange = (1, 10)")
scatter!(ax, 4, 0, color = 3, colormap = :tab10, colorrange = (1, 10))
text!(ax, 4, 0, text = "color = 3\ncolormap = :tab10\ncolorrange = (1, 10)")
text!(ax, 2.5, -1, text = "color = 1:10\ncolormap = :viridis\ncolorrange = automatic")
scatter!(ax, range(1, 4, length = 10), fill(-1, 10), color = 1:10, colormap = :viridis)
text!(ax, 2.5, -2, text = "color = [1, 2, 3, 4, NaN, 6, 7, 8, 9, 10]\ncolormap = :viridis\ncolorrange = (2, 9)")
scatter!(ax, range(1, 4, length = 10), fill(-2, 10), color = [1, 2, 3, 4, NaN, 6, 7, 8, 9, 10], colormap = :viridis, colorrange = (2, 9))
text!(ax, 2.5, -3, text = "color = [1, 2, 3, 4, NaN, 6, 7, 8, 9, 10]\ncolormap = :viridis\ncolorrange = (2, 9)\nnan_color = :red, highclip = :magenta, lowclip = :cyan")
scatter!(ax, range(1, 4, length = 10), fill(-3, 10), color = [1, 2, 3, 4, NaN, 6, 7, 8, 9, 10], colormap = :viridis, colorrange = (2, 9), nan_color = :red, highclip = :magenta, lowclip = :cyan)
text!(ax, 2.5, -4, text = "color = HSV.(range(0, 360, 10), 50, 50)")
scatter!(ax, range(1, 4, length = 10), fill(-4, 10), color = HSV.(range(0, 360, 10), 50, 50))
text!(ax, 2.5, -5, text = "color = 1:10\ncolormap = (:viridis, 0.5)\ncolorrange = automatic")
scatter!(ax, range(1, 4, length = 10), fill(-5, 10), color = 1:10, colormap = (:viridis, 0.5))
text!(ax, 2.5, -6, text = "color = 1:10\ncolormap = [:red, :orange, :brown]\ncolorrange = automatic")
scatter!(ax, range(1, 4, length = 10), fill(-6, 10), color = 1:10, colormap = [:red, :orange, :brown])
text!(ax, 2.5, -7, text = "color = 1:10\ncolormap = Reverse(:viridis)\ncolorrange = automatic")
scatter!(ax, range(1, 4, length = 10), fill(-7, 10), color = 1:10, colormap = Reverse(:viridis))
f
end

Named colors
Named colors in Makie.jl (e.g., :blue
) are parsed using Colors.jl and thus have a large array of possibilities under CSS specifications. You can find a plotted table of all possible names in this page.
Colormaps
Makie's default categorical color palette used for cycling is a reordered version of the one presented in Wong (2011).
Makie's default continuous color map is :viridis
which is a perceptually uniform colormap originally developed for matplotlib.
using CairoMakie
f, ax, sc = scatter(1:7, fill(1, 7), color = Makie.wong_colors(), markersize = 50)
hidedecorations!(ax)
hidespines!(ax)
text!(ax, 4, 1, text = "Makie.wong_colors()",
align = (:center, :bottom), offset = (0, 30))
scatter!(range(1, 7, 20), fill(0, 20), color = 1:20, markersize = 50)
text!(ax, 4, 0, text = ":viridis",
align = (:center, :bottom), offset = (0, 30))
ylims!(ax, -1, 2)
f
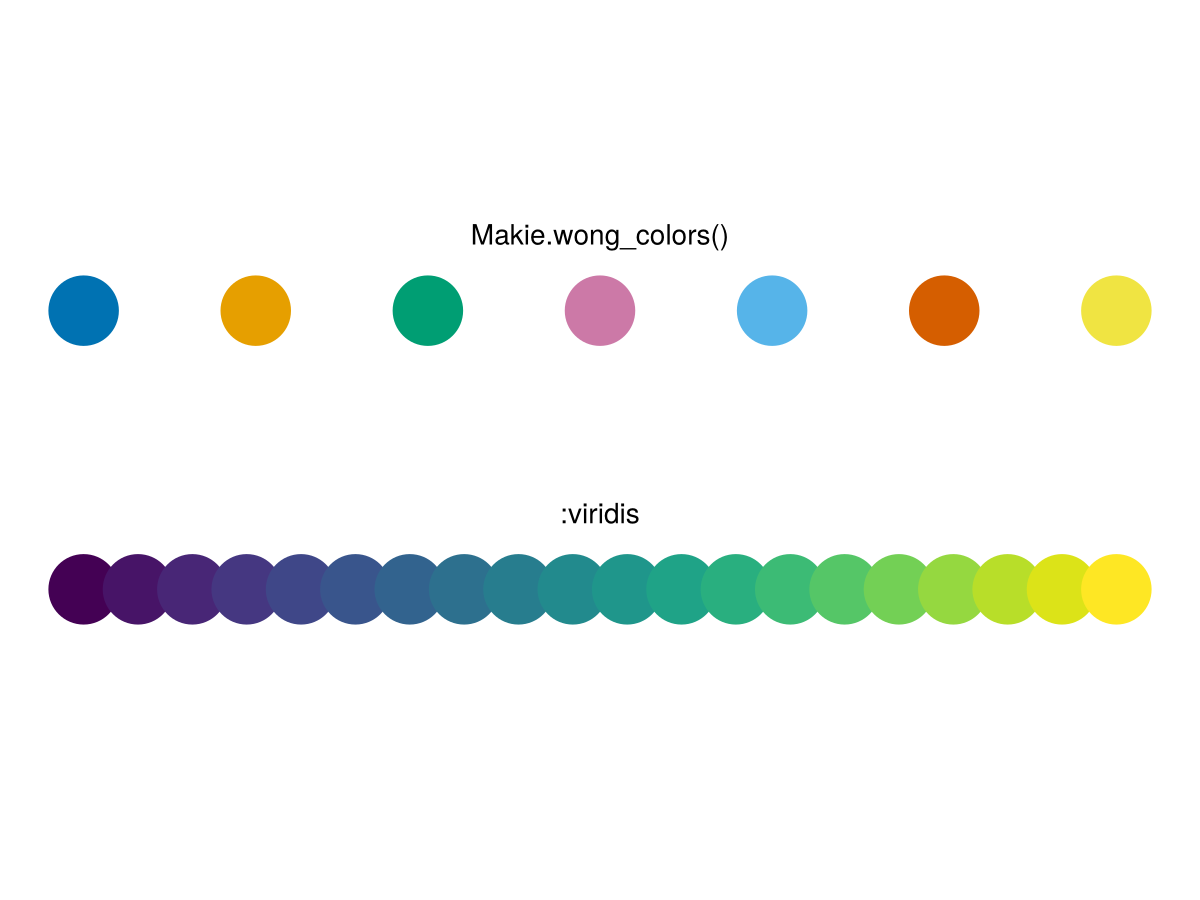
The following is a list of all the colormaps accessible via a Symbol
in Makie which are defined in ColorSchemes.jl:
misc
These colorschemes are not defined or provide different colors in ColorSchemes.jl They are kept for compatibility with the old behaviour of Makie, before v0.10.
NAME | Categorical variant | Continuous variant |
---|---|---|
:default | ||
:blues | ||
:bluesreds | ||
:darkrainbow | ||
:darktest | ||
:grays | ||
:greens | ||
:heat | ||
:lightrainbow | ||
:lighttest | ||
:rainbow | ||
:reds | ||
:redsblues |
cmocean
NAME | Categorical variant | Continuous variant |
---|---|---|
:algae | ||
:amp | ||
:balance | ||
:curl | ||
:deep | ||
:delta | ||
:dense | ||
:diff | ||
:grays | ||
:haline | ||
:ice | ||
:matter | ||
:oxy | ||
:phase | ||
:rain | ||
:solar | ||
:speed | ||
:tarn | ||
:tempo | ||
:thermal | ||
:topo | ||
:turbid |
scientific
NAME | Categorical variant | Continuous variant |
---|---|---|
:acton | ||
:acton10 | ||
:acton100 | ||
:acton25 | ||
:acton50 | ||
:actonS | ||
:bam | ||
:bam10 | ||
:bam100 | ||
:bam25 | ||
:bam50 | ||
:bamO | ||
:bamO10 | ||
:bamO100 | ||
:bamO25 | ||
:bamO50 | ||
:bamako | ||
:bamako10 | ||
:bamako100 | ||
:bamako25 | ||
:bamako50 | ||
:bamakoS | ||
:batlow | ||
:batlow10 | ||
:batlow100 | ||
:batlow25 | ||
:batlow50 | ||
:batlowK | ||
:batlowK10 | ||
:batlowK100 | ||
:batlowK25 | ||
:batlowK50 | ||
:batlowKS | ||
:batlowS | ||
:batlowW | ||
:batlowW10 | ||
:batlowW100 | ||
:batlowW25 | ||
:batlowW50 | ||
:batlowWS | ||
:berlin | ||
:berlin10 | ||
:berlin100 | ||
:berlin25 | ||
:berlin50 | ||
:bilbao | ||
:bilbao10 | ||
:bilbao100 | ||
:bilbao25 | ||
:bilbao50 | ||
:bilbaoS | ||
:broc | ||
:broc10 | ||
:broc100 | ||
:broc25 | ||
:broc50 | ||
:brocO | ||
:brocO10 | ||
:brocO100 | ||
:brocO25 | ||
:brocO50 | ||
:buda | ||
:buda10 | ||
:buda100 | ||
:buda25 | ||
:buda50 | ||
:budaS | ||
:bukavu | ||
:bukavu10 | ||
:bukavu100 | ||
:bukavu25 | ||
:bukavu50 | ||
:cork | ||
:cork10 | ||
:cork100 | ||
:cork25 | ||
:cork50 | ||
:corkO | ||
:corkO10 | ||
:corkO100 | ||
:corkO25 | ||
:corkO50 | ||
:davos | ||
:davos10 | ||
:davos100 | ||
:davos25 | ||
:davos50 | ||
:davosS | ||
:devon | ||
:devon10 | ||
:devon100 | ||
:devon25 | ||
:devon50 | ||
:devonS | ||
:fes | ||
:fes10 | ||
:fes100 | ||
:fes25 | ||
:fes50 | ||
:glasgow | ||
:glasgow10 | ||
:glasgow100 | ||
:glasgow25 | ||
:glasgow50 | ||
:glasgowS | ||
:grayC | ||
:grayC10 | ||
:grayC100 | ||
:grayC25 | ||
:grayC50 | ||
:grayCS | ||
:hawaii | ||
:hawaii10 | ||
:hawaii100 | ||
:hawaii25 | ||
:hawaii50 | ||
:hawaiiS | ||
:imola | ||
:imola10 | ||
:imola100 | ||
:imola25 | ||
:imola50 | ||
:imolaS | ||
:lajolla | ||
:lajolla10 | ||
:lajolla100 | ||
:lajolla25 | ||
:lajolla50 | ||
:lajollaS | ||
:lapaz | ||
:lapaz10 | ||
:lapaz100 | ||
:lapaz25 | ||
:lapaz50 | ||
:lapazS | ||
:lipari | ||
:lipari10 | ||
:lipari100 | ||
:lipari25 | ||
:lipari50 | ||
:lipariS | ||
:lisbon | ||
:lisbon10 | ||
:lisbon100 | ||
:lisbon25 | ||
:lisbon50 | ||
:managua | ||
:managua10 | ||
:managua100 | ||
:managua25 | ||
:managua50 | ||
:navia | ||
:navia10 | ||
:navia100 | ||
:navia25 | ||
:navia50 | ||
:naviaS | ||
:nuuk | ||
:nuuk10 | ||
:nuuk100 | ||
:nuuk25 | ||
:nuuk50 | ||
:nuukS | ||
:oleron | ||
:oleron10 | ||
:oleron100 | ||
:oleron25 | ||
:oleron50 | ||
:oslo | ||
:oslo10 | ||
:oslo100 | ||
:oslo25 | ||
:oslo50 | ||
:osloS | ||
:roma | ||
:roma10 | ||
:roma100 | ||
:roma25 | ||
:roma50 | ||
:romaO | ||
:romaO10 | ||
:romaO100 | ||
:romaO25 | ||
:romaO50 | ||
:tofino | ||
:tofino10 | ||
:tofino100 | ||
:tofino25 | ||
:tofino50 | ||
:tokyo | ||
:tokyo10 | ||
:tokyo100 | ||
:tokyo25 | ||
:tokyo50 | ||
:tokyoS | ||
:turku | ||
:turku10 | ||
:turku100 | ||
:turku25 | ||
:turku50 | ||
:turkuS | ||
:vanimo | ||
:vanimo10 | ||
:vanimo100 | ||
:vanimo25 | ||
:vanimo50 | ||
:vik | ||
:vik10 | ||
:vik100 | ||
:vik25 | ||
:vik50 | ||
:vikO | ||
:vikO10 | ||
:vikO100 | ||
:vikO25 | ||
:vikO50 |
matplotlib
NAME | Categorical variant | Continuous variant |
---|---|---|
:Wistia | ||
:autumn1 | ||
:binary | ||
:bone | ||
:bone_1 | ||
:brg | ||
:bwr | ||
:cool | ||
:coolwarm | ||
:copper | ||
:flag | ||
:gist_earth | ||
:gist_gray | ||
:gist_heat | ||
:gist_ncar | ||
:gist_rainbow | ||
:gist_stern | ||
:gist_yarg | ||
:gray1 | ||
:hot | ||
:hsv | ||
:inferno | ||
:jet1 | ||
:magma | ||
:nipy_spectral | ||
:pink | ||
:plasma | ||
:prism | ||
:seismic | ||
:spring | ||
:summer | ||
:tab10 | ||
:tab20 | ||
:tab20b | ||
:tab20c | ||
:terrain | ||
:twilight | ||
:viridis | ||
:winter |
colorbrewer
NAME | Categorical variant | Continuous variant |
---|---|---|
:Accent_3 | ||
:Accent_4 | ||
:Accent_5 | ||
:Accent_6 | ||
:Accent_7 | ||
:Accent_8 | ||
:Blues | ||
:Blues_3 | ||
:Blues_4 | ||
:Blues_5 | ||
:Blues_6 | ||
:Blues_7 | ||
:Blues_8 | ||
:Blues_9 | ||
:BrBG_10 | ||
:BrBG_11 | ||
:BrBG_3 | ||
:BrBG_4 | ||
:BrBG_5 | ||
:BrBG_6 | ||
:BrBG_7 | ||
:BrBG_8 | ||
:BrBG_9 | ||
:BrBg | ||
:BuGn | ||
:BuGn_3 | ||
:BuGn_4 | ||
:BuGn_5 | ||
:BuGn_6 | ||
:BuGn_7 | ||
:BuGn_8 | ||
:BuGn_9 | ||
:BuPu | ||
:BuPu_3 | ||
:BuPu_4 | ||
:BuPu_5 | ||
:BuPu_6 | ||
:BuPu_7 | ||
:BuPu_8 | ||
:BuPu_9 | ||
:Dark2_3 | ||
:Dark2_4 | ||
:Dark2_5 | ||
:Dark2_6 | ||
:Dark2_7 | ||
:Dark2_8 | ||
:GnBu | ||
:GnBu_3 | ||
:GnBu_4 | ||
:GnBu_5 | ||
:GnBu_6 | ||
:GnBu_7 | ||
:GnBu_8 | ||
:GnBu_9 | ||
:Greens | ||
:Greens_3 | ||
:Greens_4 | ||
:Greens_5 | ||
:Greens_6 | ||
:Greens_7 | ||
:Greens_8 | ||
:Greens_9 | ||
:Greys | ||
:Greys_3 | ||
:Greys_4 | ||
:Greys_5 | ||
:Greys_6 | ||
:Greys_7 | ||
:Greys_8 | ||
:Greys_9 | ||
:OrRd | ||
:OrRd_3 | ||
:OrRd_4 | ||
:OrRd_5 | ||
:OrRd_6 | ||
:OrRd_7 | ||
:OrRd_8 | ||
:OrRd_9 | ||
:Oranges | ||
:Oranges_3 | ||
:Oranges_4 | ||
:Oranges_5 | ||
:Oranges_6 | ||
:Oranges_7 | ||
:Oranges_8 | ||
:Oranges_9 | ||
:PRGn | ||
:PRGn_10 | ||
:PRGn_11 | ||
:PRGn_3 | ||
:PRGn_4 | ||
:PRGn_5 | ||
:PRGn_6 | ||
:PRGn_7 | ||
:PRGn_8 | ||
:PRGn_9 | ||
:Paired_10 | ||
:Paired_11 | ||
:Paired_12 | ||
:Paired_3 | ||
:Paired_4 | ||
:Paired_5 | ||
:Paired_6 | ||
:Paired_7 | ||
:Paired_8 | ||
:Paired_9 | ||
:Pastel1_3 | ||
:Pastel1_4 | ||
:Pastel1_5 | ||
:Pastel1_6 | ||
:Pastel1_7 | ||
:Pastel1_8 | ||
:Pastel1_9 | ||
:Pastel2_3 | ||
:Pastel2_4 | ||
:Pastel2_5 | ||
:Pastel2_6 | ||
:Pastel2_7 | ||
:Pastel2_8 | ||
:PiYG | ||
:PiYG_10 | ||
:PiYG_11 | ||
:PiYG_3 | ||
:PiYG_4 | ||
:PiYG_5 | ||
:PiYG_6 | ||
:PiYG_7 | ||
:PiYG_8 | ||
:PiYG_9 | ||
:PuBu | ||
:PuBuGn | ||
:PuBuGn_3 | ||
:PuBuGn_4 | ||
:PuBuGn_5 | ||
:PuBuGn_6 | ||
:PuBuGn_7 | ||
:PuBuGn_8 | ||
:PuBuGn_9 | ||
:PuBu_3 | ||
:PuBu_4 | ||
:PuBu_5 | ||
:PuBu_6 | ||
:PuBu_7 | ||
:PuBu_8 | ||
:PuBu_9 | ||
:PuOr | ||
:PuOr_10 | ||
:PuOr_11 | ||
:PuOr_3 | ||
:PuOr_4 | ||
:PuOr_5 | ||
:PuOr_6 | ||
:PuOr_7 | ||
:PuOr_8 | ||
:PuOr_9 | ||
:PuRd | ||
:PuRd_3 | ||
:PuRd_4 | ||
:PuRd_5 | ||
:PuRd_6 | ||
:PuRd_7 | ||
:PuRd_8 | ||
:PuRd_9 | ||
:Purples | ||
:Purples_3 | ||
:Purples_4 | ||
:Purples_5 | ||
:Purples_6 | ||
:Purples_7 | ||
:Purples_8 | ||
:Purples_9 | ||
:RdBu | ||
:RdBu_10 | ||
:RdBu_11 | ||
:RdBu_3 | ||
:RdBu_4 | ||
:RdBu_5 | ||
:RdBu_6 | ||
:RdBu_7 | ||
:RdBu_8 | ||
:RdBu_9 | ||
:RdGy | ||
:RdGy_10 | ||
:RdGy_11 | ||
:RdGy_3 | ||
:RdGy_4 | ||
:RdGy_5 | ||
:RdGy_6 | ||
:RdGy_7 | ||
:RdGy_8 | ||
:RdGy_9 | ||
:RdPu | ||
:RdPu_3 | ||
:RdPu_4 | ||
:RdPu_5 | ||
:RdPu_6 | ||
:RdPu_7 | ||
:RdPu_8 | ||
:RdPu_9 | ||
:RdYlBu | ||
:RdYlBu_10 | ||
:RdYlBu_11 | ||
:RdYlBu_3 | ||
:RdYlBu_4 | ||
:RdYlBu_5 | ||
:RdYlBu_6 | ||
:RdYlBu_7 | ||
:RdYlBu_8 | ||
:RdYlBu_9 | ||
:RdYlGn | ||
:RdYlGn_10 | ||
:RdYlGn_11 | ||
:RdYlGn_3 | ||
:RdYlGn_4 | ||
:RdYlGn_5 | ||
:RdYlGn_6 | ||
:RdYlGn_7 | ||
:RdYlGn_8 | ||
:RdYlGn_9 | ||
:Reds | ||
:Reds_3 | ||
:Reds_4 | ||
:Reds_5 | ||
:Reds_6 | ||
:Reds_7 | ||
:Reds_8 | ||
:Reds_9 | ||
:Set1_3 | ||
:Set1_4 | ||
:Set1_5 | ||
:Set1_6 | ||
:Set1_7 | ||
:Set1_8 | ||
:Set1_9 | ||
:Set2_3 | ||
:Set2_4 | ||
:Set2_5 | ||
:Set2_6 | ||
:Set2_7 | ||
:Set2_8 | ||
:Set3_10 | ||
:Set3_11 | ||
:Set3_12 | ||
:Set3_3 | ||
:Set3_4 | ||
:Set3_5 | ||
:Set3_6 | ||
:Set3_7 | ||
:Set3_8 | ||
:Set3_9 | ||
:Spectral | ||
:Spectral_10 | ||
:Spectral_11 | ||
:Spectral_3 | ||
:Spectral_4 | ||
:Spectral_5 | ||
:Spectral_6 | ||
:Spectral_7 | ||
:Spectral_8 | ||
:Spectral_9 | ||
:YlGn | ||
:YlGnBu | ||
:YlGnBu_3 | ||
:YlGnBu_4 | ||
:YlGnBu_5 | ||
:YlGnBu_6 | ||
:YlGnBu_7 | ||
:YlGnBu_8 | ||
:YlGnBu_9 | ||
:YlGn_3 | ||
:YlGn_4 | ||
:YlGn_5 | ||
:YlGn_6 | ||
:YlGn_7 | ||
:YlGn_8 | ||
:YlGn_9 | ||
:YlOrBr | ||
:YlOrBr_3 | ||
:YlOrBr_4 | ||
:YlOrBr_5 | ||
:YlOrBr_6 | ||
:YlOrBr_7 | ||
:YlOrBr_8 | ||
:YlOrBr_9 | ||
:YlOrRd | ||
:YlOrRd_3 | ||
:YlOrRd_4 | ||
:YlOrRd_5 | ||
:YlOrRd_6 | ||
:YlOrRd_7 | ||
:YlOrRd_8 | ||
:YlOrRd_9 |
gnuplot
NAME | Categorical variant | Continuous variant |
---|---|---|
:afmhot | ||
:gnuplot | ||
:gnuplot2 | ||
:ocean | ||
:rainbow1 |
colorcet
NAME | Categorical variant | Continuous variant |
---|---|---|
:cyclic_grey_15_85_c0_n256 | ||
:cyclic_grey_15_85_c0_n256_s25 | ||
:cyclic_mrybm_35_75_c68_n256 | ||
:cyclic_mrybm_35_75_c68_n256_s25 | ||
:cyclic_mygbm_30_95_c78_n256 | ||
:cyclic_mygbm_30_95_c78_n256_s25 | ||
:cyclic_protanopic_deuteranopic_bwyk_16_96_c31_n256 | ||
:cyclic_protanopic_deuteranopic_wywb_55_96_c33_n256 | ||
:cyclic_tritanopic_cwrk_40_100_c20_n256 | ||
:cyclic_tritanopic_wrwc_70_100_c20_n256 | ||
:cyclic_wrwbw_40_90_c42_n256 | ||
:cyclic_wrwbw_40_90_c42_n256_s25 | ||
:diverging_bkr_55_10_c35_n256 | ||
:diverging_bky_60_10_c30_n256 | ||
:diverging_bwg_20_95_c41_n256 | ||
:diverging_bwr_20_95_c54_n256 | ||
:diverging_bwr_40_95_c42_n256 | ||
:diverging_bwr_55_98_c37_n256 | ||
:diverging_cwm_80_100_c22_n256 | ||
:diverging_gkr_60_10_c40_n256 | ||
:diverging_gwr_55_95_c38_n256 | ||
:diverging_gwv_55_95_c39_n256 | ||
:diverging_isoluminant_cjm_75_c23_n256 | ||
:diverging_isoluminant_cjm_75_c24_n256 | ||
:diverging_isoluminant_cjo_70_c25_n256 | ||
:diverging_linear_bjr_30_55_c53_n256 | ||
:diverging_linear_bjy_30_90_c45_n256 | ||
:diverging_protanopic_deuteranopic_bwy_60_95_c32_n256 | ||
:diverging_rainbow_bgymr_45_85_c67_n256 | ||
:diverging_tritanopic_cwr_75_98_c20_n256 | ||
:glasbey_bw_minc_20_hue_150_280_n256 | ||
:glasbey_bw_minc_20_hue_330_100_n256 | ||
:glasbey_bw_minc_20_maxl_70_n256 | ||
:glasbey_bw_minc_20_minl_30_n256 | ||
:glasbey_bw_minc_20_n256 | ||
:glasbey_bw_n256 | ||
:glasbey_category10_n256 | ||
:glasbey_hv_n256 | ||
:isoluminant_cgo_70_c39_n256 | ||
:isoluminant_cgo_80_c38_n256 | ||
:isoluminant_cm_70_c39_n256 | ||
:linear_bgy_10_95_c74_n256 | ||
:linear_bgyw_15_100_c67_n256 | ||
:linear_bgyw_15_100_c68_n256 | ||
:linear_bgyw_20_98_c66_n256 | ||
:linear_blue_5_95_c73_n256 | ||
:linear_blue_95_50_c20_n256 | ||
:linear_bmw_5_95_c86_n256 | ||
:linear_bmw_5_95_c89_n256 | ||
:linear_bmy_10_95_c71_n256 | ||
:linear_bmy_10_95_c78_n256 | ||
:linear_gow_60_85_c27_n256 | ||
:linear_gow_65_90_c35_n256 | ||
:linear_green_5_95_c69_n256 | ||
:linear_grey_0_100_c0_n256 | ||
:linear_grey_10_95_c0_n256 | ||
:linear_kbc_5_95_c73_n256 | ||
:linear_kbgyw_5_98_c62_n256 | ||
:linear_kgy_5_95_c69_n256 | ||
:linear_kry_0_97_c73_n256 | ||
:linear_kry_5_95_c72_n256 | ||
:linear_kry_5_98_c75_n256 | ||
:linear_kryw_0_100_c71_n256 | ||
:linear_kryw_5_100_c64_n256 | ||
:linear_kryw_5_100_c67_n256 | ||
:linear_protanopic_deuteranopic_kbjyw_5_95_c25_n256 | ||
:linear_protanopic_deuteranopic_kbw_5_98_c40_n256 | ||
:linear_ternary_blue_0_44_c57_n256 | ||
:linear_ternary_green_0_46_c42_n256 | ||
:linear_ternary_red_0_50_c52_n256 | ||
:linear_tritanopic_krjcw_5_95_c24_n256 | ||
:linear_tritanopic_krjcw_5_98_c46_n256 | ||
:linear_wcmr_100_45_c42_n256 | ||
:linear_worb_100_25_c53_n256 | ||
:linear_wyor_100_45_c55_n256 | ||
:rainbow_bgyr_35_85_c72_n256 | ||
:rainbow_bgyr_35_85_c73_n256 | ||
:rainbow_bgyrm_35_85_c69_n256 | ||
:rainbow_bgyrm_35_85_c71_n256 |
seaborn
NAME | Categorical variant | Continuous variant |
---|---|---|
:seaborn_bright | ||
:seaborn_bright6 | ||
:seaborn_colorblind | ||
:seaborn_colorblind6 | ||
:seaborn_crest_gradient | ||
:seaborn_dark | ||
:seaborn_dark6 | ||
:seaborn_deep | ||
:seaborn_deep6 | ||
:seaborn_flare_gradient | ||
:seaborn_icefire_gradient | ||
:seaborn_mako_gradient | ||
:seaborn_muted | ||
:seaborn_muted6 | ||
:seaborn_pastel | ||
:seaborn_pastel6 | ||
:seaborn_rocket_gradient |
general
NAME | Categorical variant | Continuous variant |
---|---|---|
:CMRmap | ||
:alpine | ||
:aquamarine | ||
:army | ||
:atlantic | ||
:auerbach | ||
:aurora | ||
:autumn | ||
:avocado | ||
:beach | ||
:blackbody | ||
:bluegreenyellow | ||
:bosch_garden | ||
:bosch_hell | ||
:botticelli | ||
:brass | ||
:browncyan | ||
:canaletto | ||
:candy | ||
:cezanne | ||
:cherry | ||
:cividis | ||
:cmyk | ||
:coffee | ||
:cubehelix | ||
:darkrainbow | ||
:darkterrain | ||
:deepsea | ||
:dracula | ||
:fall | ||
:fastie | ||
:fruitpunch | ||
:fuchsia | ||
:gold | ||
:grays1 | ||
:grayyellow | ||
:greenbrownterrain | ||
:greenpink | ||
:hokusai | ||
:holbein | ||
:island | ||
:jet | ||
:julia_colorscheme | ||
:klimt | ||
:lake | ||
:leonardo | ||
:lighttemperaturemap | ||
:lightterrain | ||
:mint | ||
:munch | ||
:neon | ||
:pastel | ||
:pearl | ||
:picasso | ||
:pigeon | ||
:plum | ||
:rainbow | ||
:redblue | ||
:redgreensplit | ||
:rembrandt | ||
:rose | ||
:rust | ||
:sandyterrain | ||
:sienna | ||
:southwest | ||
:starrynight | ||
:sun | ||
:sunset | ||
:temperaturemap | ||
:thermometer | ||
:turbo | ||
:twelvebitrainbow | ||
:valentine | ||
:vangogh | ||
:vanhelsing | ||
:vermeer | ||
:watermelon | ||
:websafe |