barplot
Makie.barplot Function
barplot(positions, heights; kwargs...)
Plots a barplot.
Plot type
The plot type alias for the barplot
function is BarPlot
.
Examples
using CairoMakie
f = Figure()
Axis(f[1, 1])
xs = 1:0.2:10
ys = 0.5 .* sin.(xs)
barplot!(xs, ys, color = :red, strokecolor = :black, strokewidth = 1)
barplot!(xs, ys .- 1, fillto = -1, color = xs, strokecolor = :black, strokewidth = 1)
f
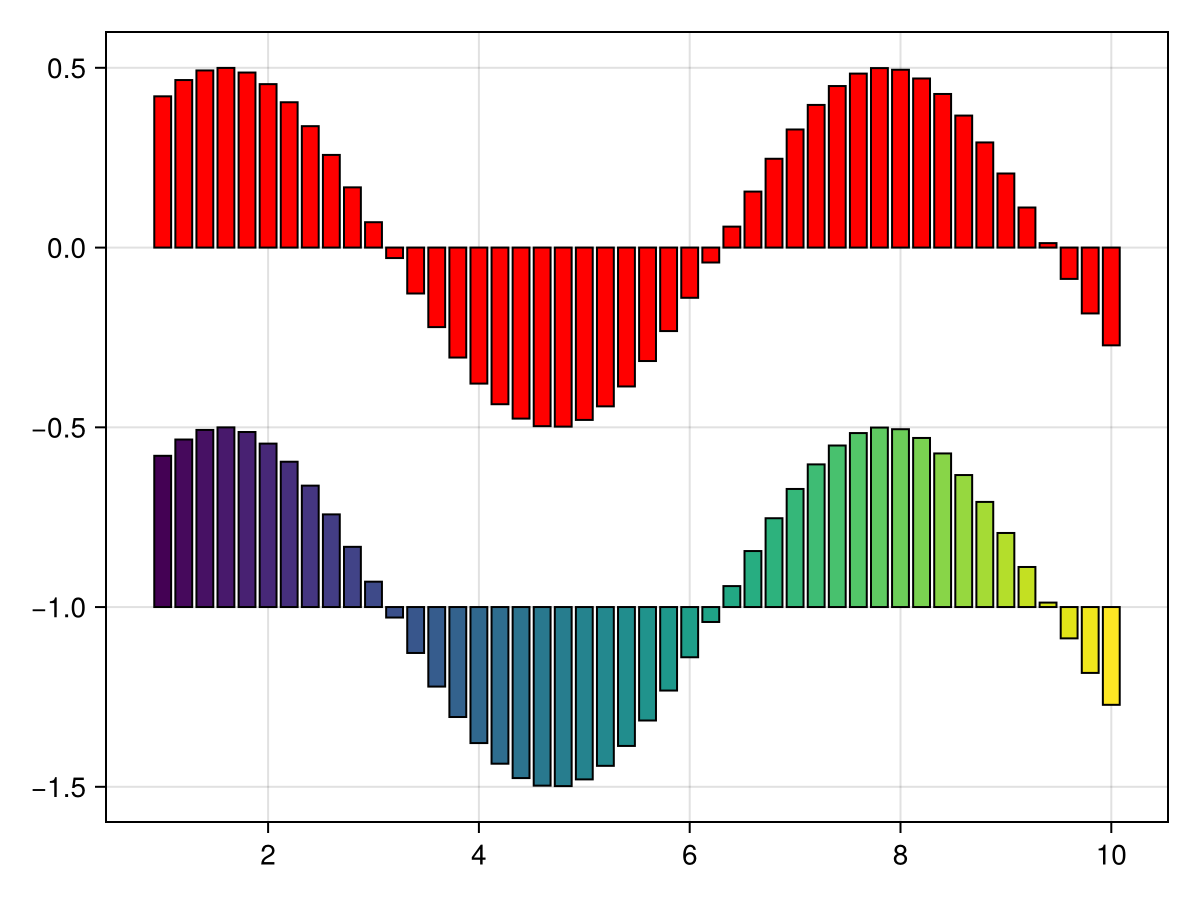
using CairoMakie
xs = 1:0.2:10
ys = 0.5 .* sin.(xs)
barplot(xs, ys, gap = 0, color = :gray85, strokecolor = :black, strokewidth = 1)
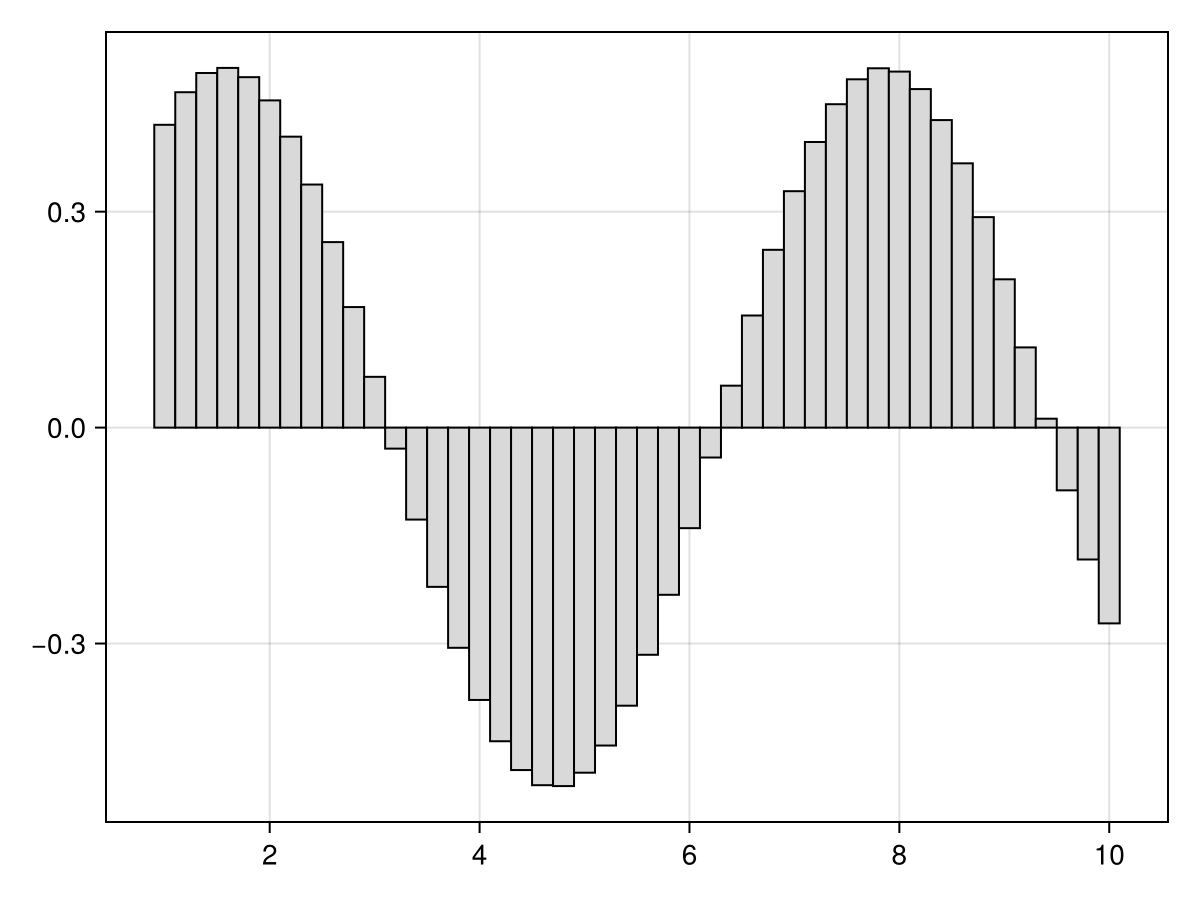
using CairoMakie
tbl = (cat = [1, 1, 1, 2, 2, 2, 3, 3, 3],
height = 0.1:0.1:0.9,
grp = [1, 2, 3, 1, 2, 3, 1, 2, 3],
grp1 = [1, 2, 2, 1, 1, 2, 1, 1, 2],
grp2 = [1, 1, 2, 1, 2, 1, 1, 2, 1]
)
barplot(tbl.cat, tbl.height,
stack = tbl.grp,
color = tbl.grp,
axis = (xticks = (1:3, ["left", "middle", "right"]),
title = "Stacked bars"),
)
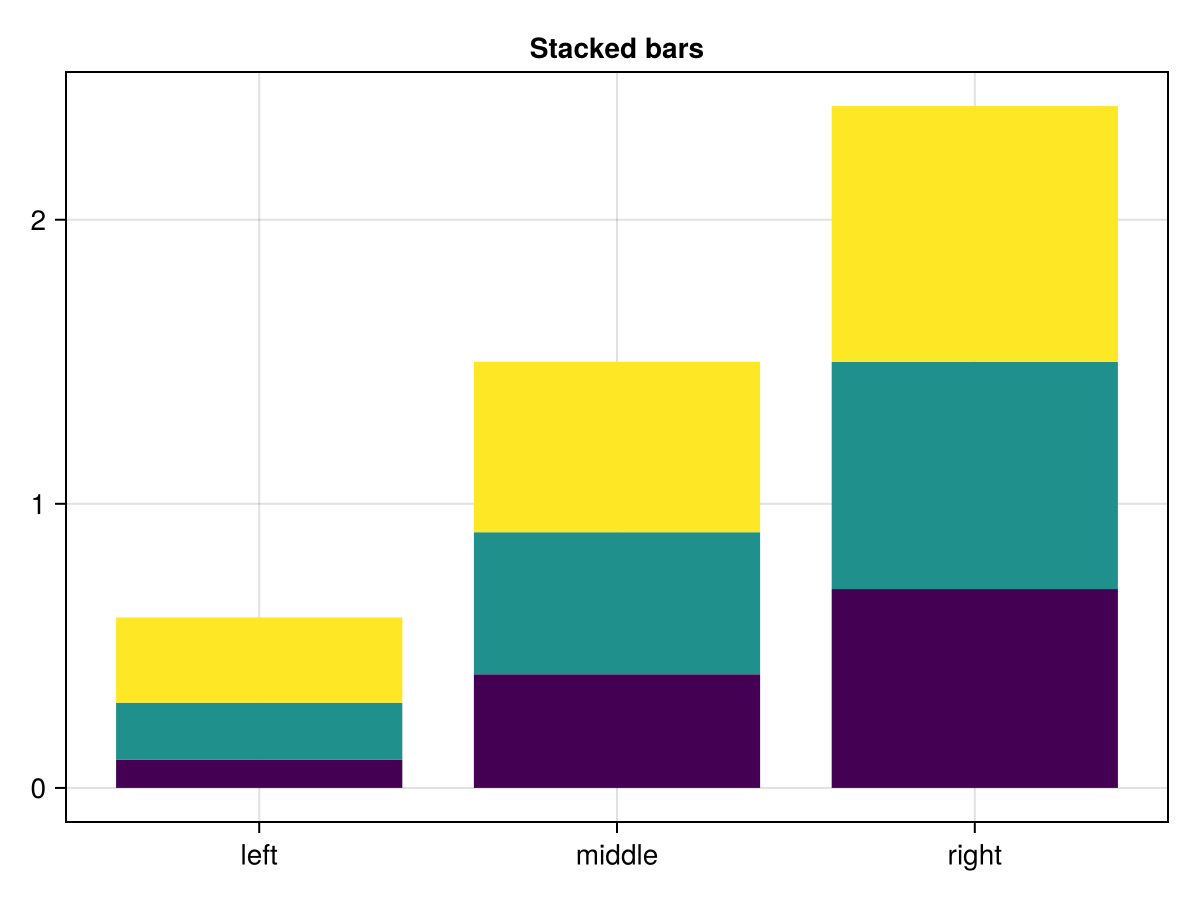
barplot(tbl.cat, tbl.height,
dodge = tbl.grp,
color = tbl.grp,
axis = (xticks = (1:3, ["left", "middle", "right"]),
title = "Dodged bars"),
)
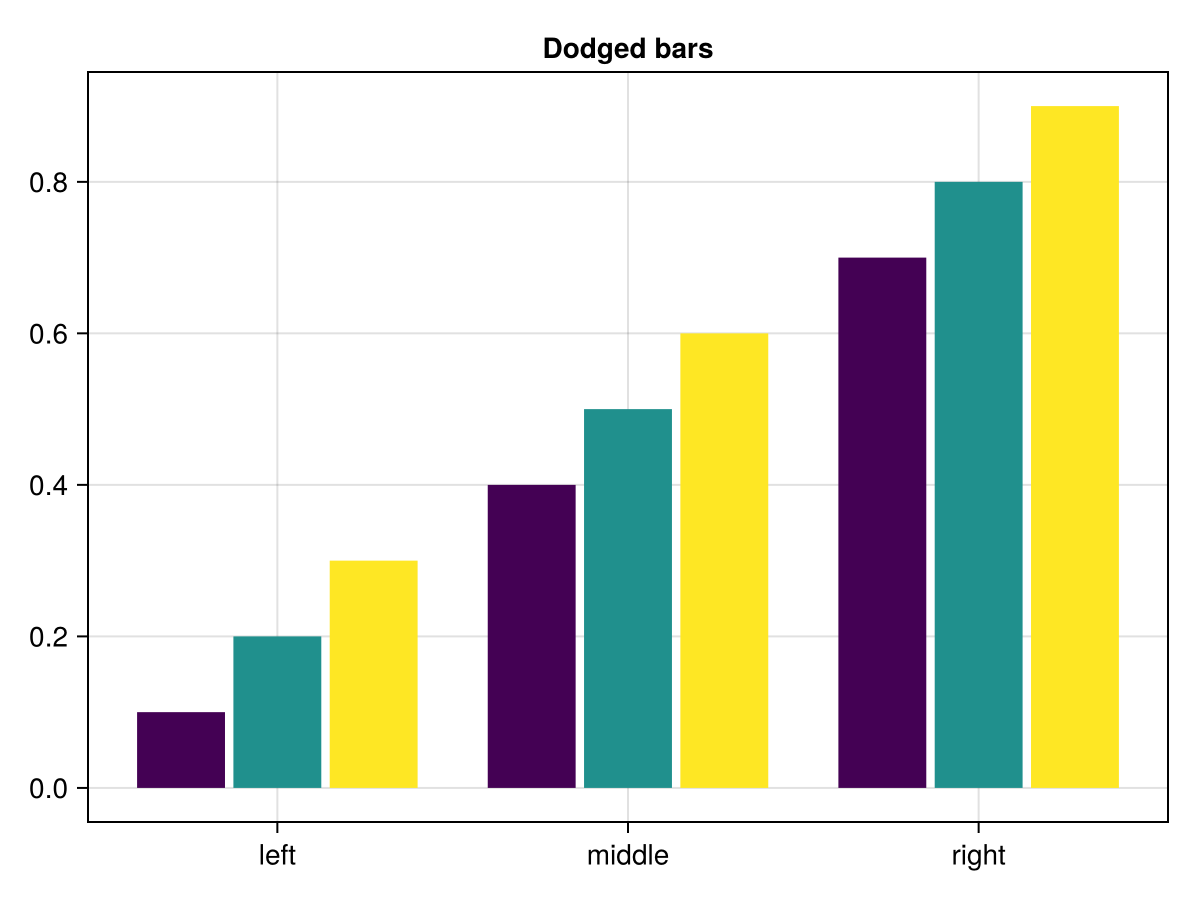
barplot(tbl.cat, tbl.height,
dodge = tbl.grp1,
stack = tbl.grp2,
color = tbl.grp,
axis = (xticks = (1:3, ["left", "middle", "right"]),
title = "Dodged and stacked bars"),
)
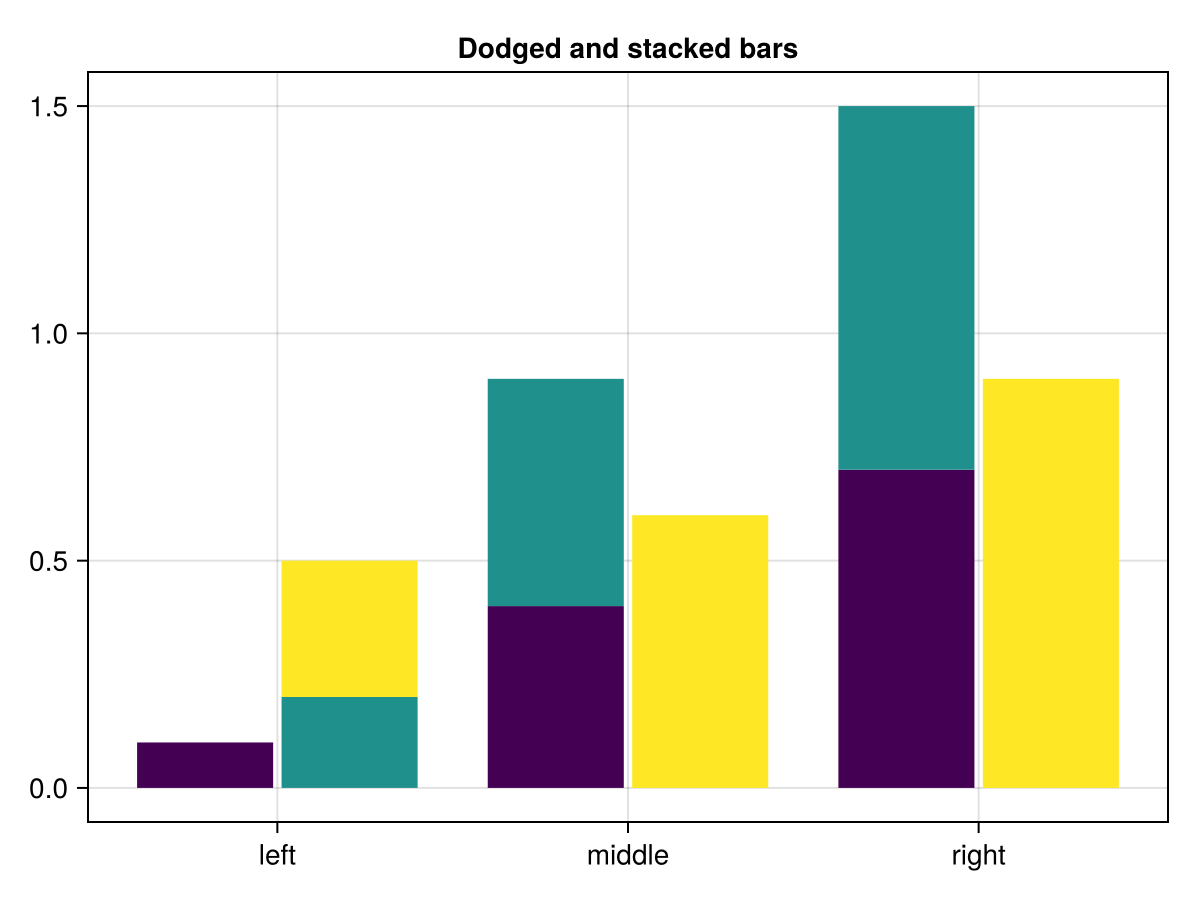
colors = Makie.wong_colors()
# Figure and Axis
fig = Figure()
ax = Axis(fig[1,1], xticks = (1:3, ["left", "middle", "right"]),
title = "Dodged bars with legend")
# Plot
barplot!(ax, tbl.cat, tbl.height,
dodge = tbl.grp,
color = colors[tbl.grp])
# Legend
labels = ["group 1", "group 2", "group 3"]
elements = [PolyElement(polycolor = colors[i]) for i in 1:length(labels)]
title = "Groups"
Legend(fig[1,2], elements, labels, title)
fig
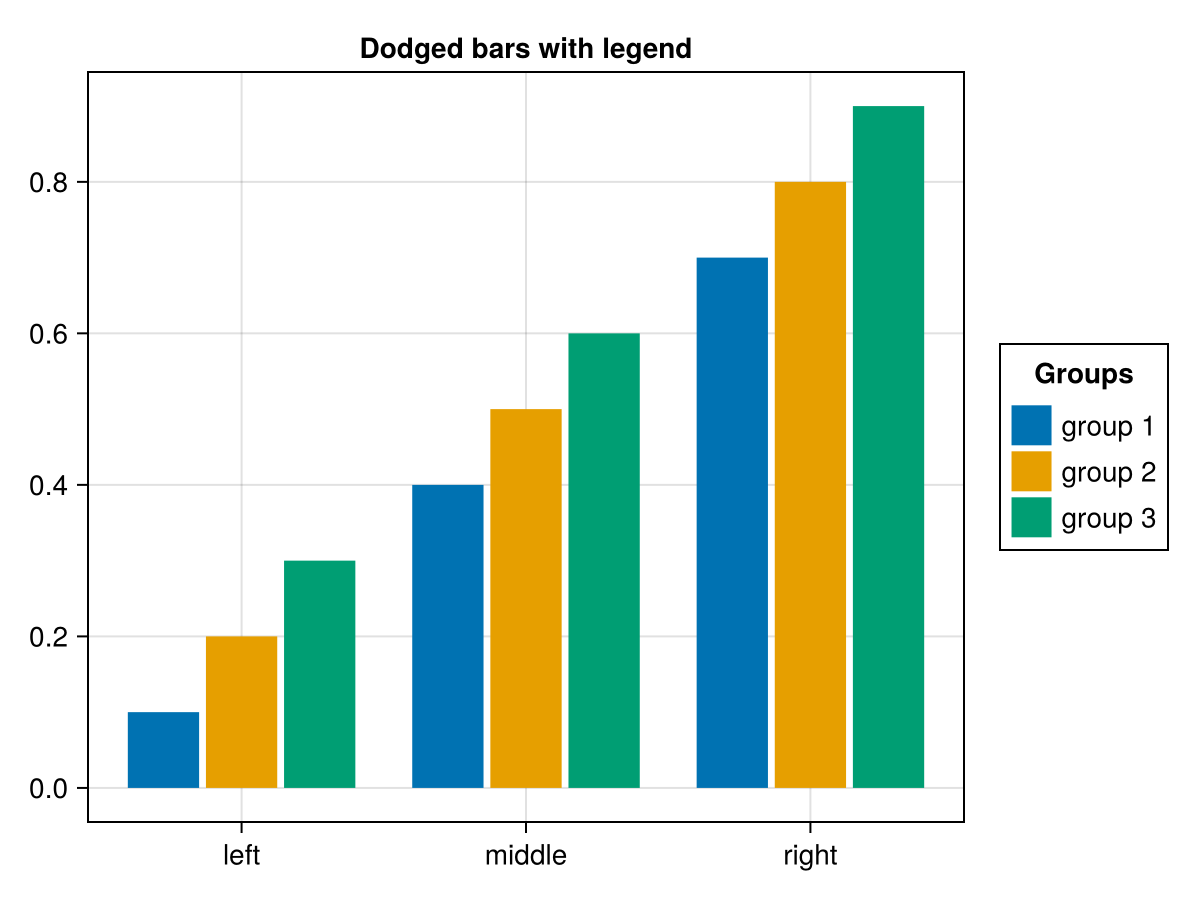
barplot(
tbl.cat, tbl.height,
dodge = tbl.grp,
color = tbl.grp,
bar_labels = :y,
axis = (xticks = (1:3, ["left", "middle", "right"]),
title = "Dodged bars horizontal with labels"),
colormap = [:red, :green, :blue],
color_over_background=:red,
color_over_bar=:white,
flip_labels_at=0.85,
direction=:x,
)
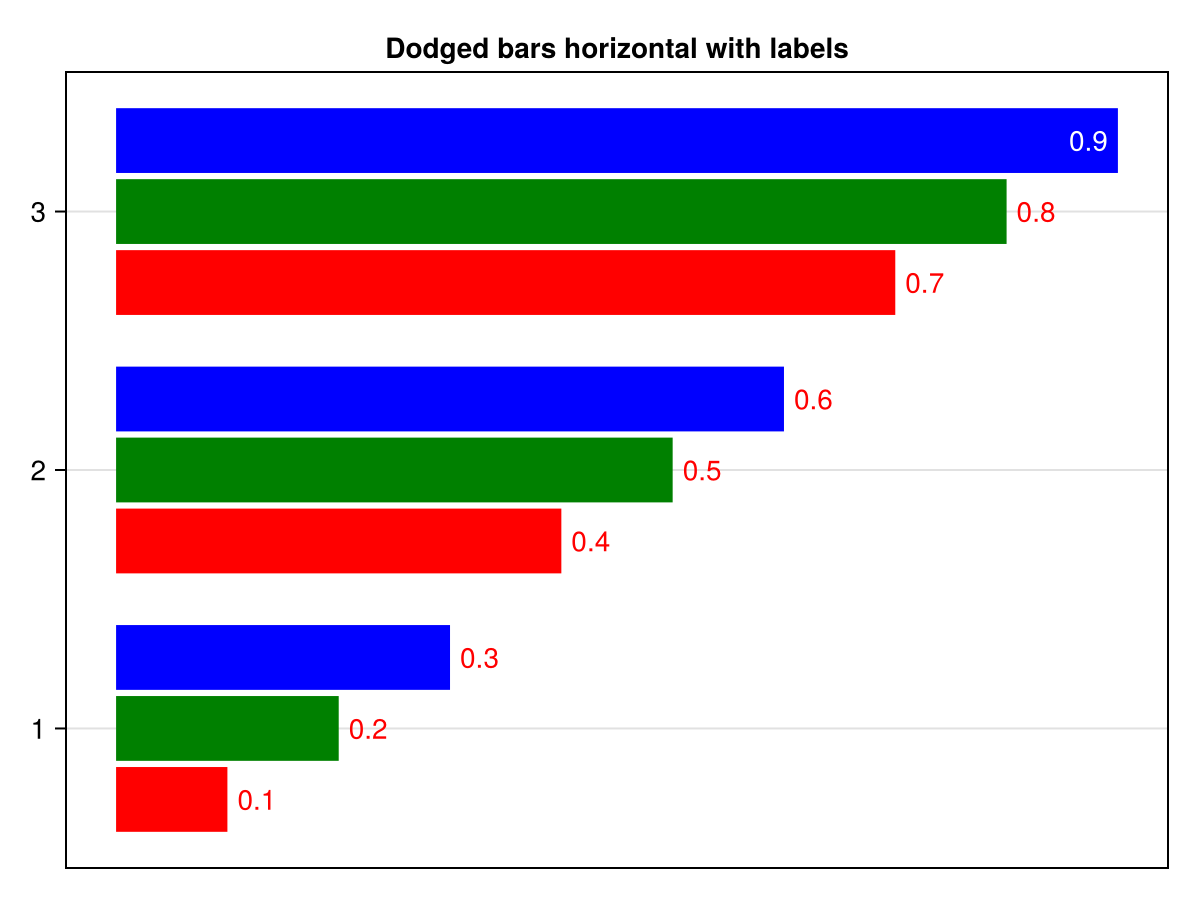
using CairoMakie
barplot([-1, -0.5, 0.5, 1],
bar_labels = :y,
axis = (title="Fonts + flip_labels_at",),
label_size = 20,
flip_labels_at=(-0.8, 0.8),
label_color=[:white, :green, :black, :white],
label_formatter = x-> "Flip at $(x)?",
label_offset = 10
)
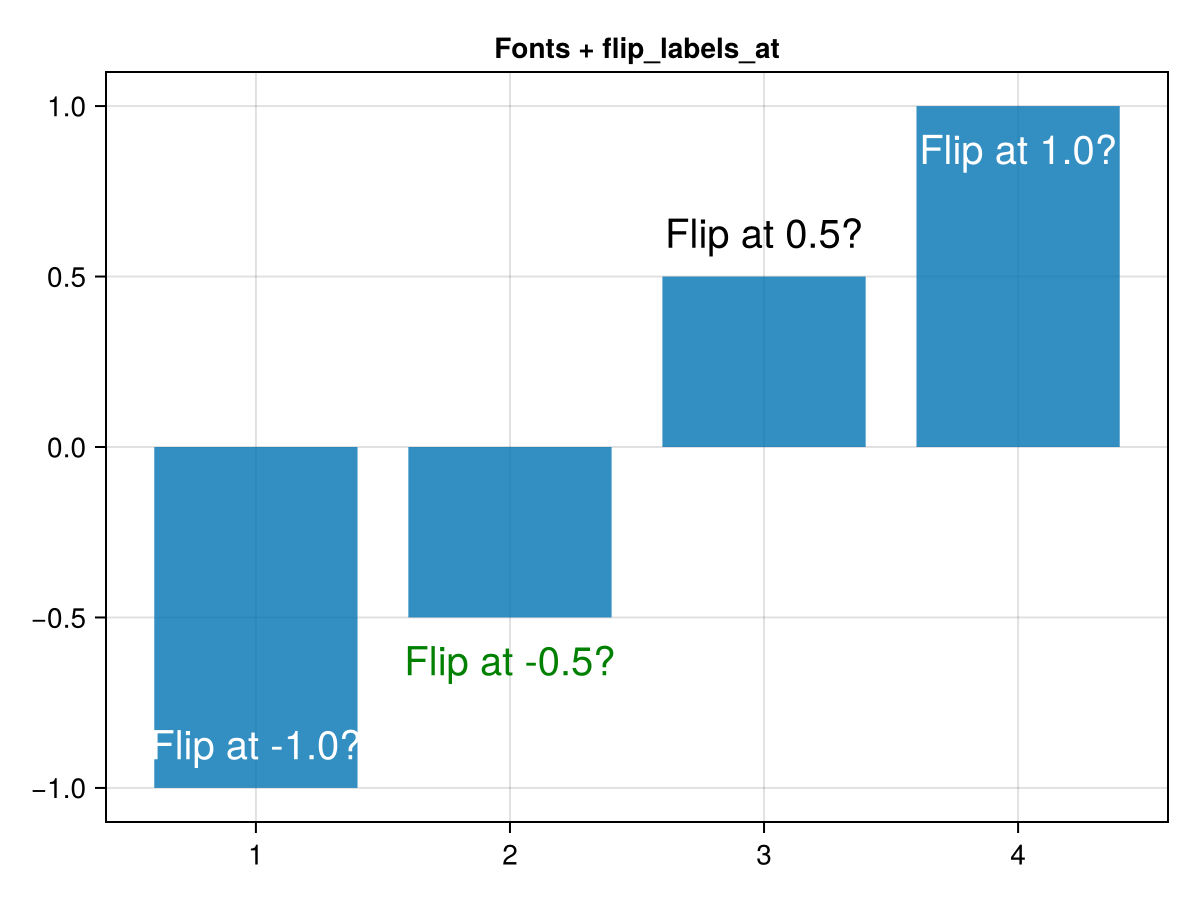
using CairoMakie
gantt = (
machine = [1, 2, 1, 2],
job = [1, 1, 2, 3],
task = [1, 2, 3, 3],
start = [1, 3, 3.5, 5],
stop = [3, 4, 5, 6]
)
fig = Figure()
ax = Axis(
fig[2,1],
yticks = (1:2, ["A","B"]),
ylabel = "Machine",
xlabel = "Time"
)
xlims!(ax, 0, maximum(gantt.stop))
cmap = Makie.to_colormap(:tab10)
barplot!(
gantt.machine,
gantt.stop,
fillto = gantt.start,
direction = :x,
color = gantt.job,
colormap = cmap,
colorrange = (1, length(cmap)),
gap = 0.5,
bar_labels = ["task #$i" for i in gantt.task],
label_position = :center,
label_color = :white,
label = ["job #$i" => (; color = i) for i in unique(gantt.job)]
)
Legend(fig[1,1], ax, "Jobs", orientation=:horizontal, tellwidth = false, tellheight = true)
fig
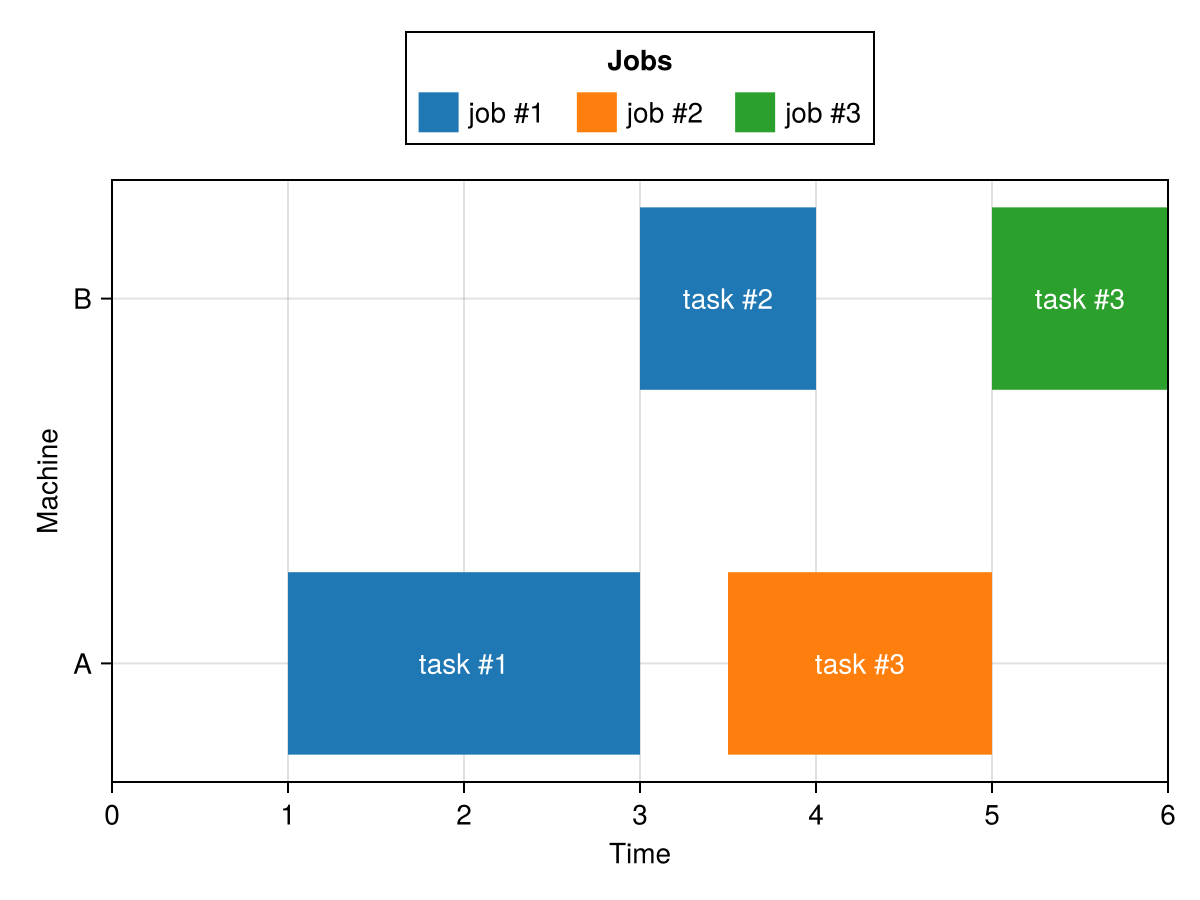
Attributes
alpha
Defaults to 1.0
The alpha value of the colormap or color attribute. Multiple alphas like in plot(alpha=0.2, color=(:red, 0.5)
, will get multiplied.
bar_labels
Defaults to nothing
Labels added at the end of each bar.
clip_planes
Defaults to automatic
Clip planes offer a way to do clipping in 3D space. You can set a Vector of up to 8 Plane3f
planes here, behind which plots will be clipped (i.e. become invisible). By default clip planes are inherited from the parent plot or scene. You can remove parent clip_planes
by passing Plane3f[]
.
color
Defaults to @inherit patchcolor
No docs available.
color_over_background
Defaults to automatic
No docs available.
color_over_bar
Defaults to automatic
No docs available.
colormap
Defaults to @inherit colormap :viridis
Sets the colormap that is sampled for numeric color
s. PlotUtils.cgrad(...)
, Makie.Reverse(any_colormap)
can be used as well, or any symbol from ColorBrewer or PlotUtils. To see all available color gradients, you can call Makie.available_gradients()
.
colorrange
Defaults to automatic
The values representing the start and end points of colormap
.
colorscale
Defaults to identity
The color transform function. Can be any function, but only works well together with Colorbar
for identity
, log
, log2
, log10
, sqrt
, logit
, Makie.pseudolog10
and Makie.Symlog10
.
cycle
Defaults to [:color => :patchcolor]
No docs available.
depth_shift
Defaults to 0.0
Adjusts the depth value of a plot after all other transformations, i.e. in clip space, where -1 <= depth <= 1
. This only applies to GLMakie and WGLMakie and can be used to adjust render order (like a tunable overdraw).
direction
Defaults to :y
Controls the direction of the bars, can be :y
(vertical) or :x
(horizontal).
dodge
Defaults to automatic
No docs available.
dodge_gap
Defaults to 0.03
No docs available.
fillto
Defaults to automatic
Controls the baseline of the bars. This is zero in the default automatic
case unless the barplot is in a log-scaled Axis
. With a log scale, the automatic default is half the minimum value because zero is an invalid value for a log scale.
flip_labels_at
Defaults to Inf
No docs available.
fxaa
Defaults to true
Adjusts whether the plot is rendered with fxaa (anti-aliasing, GLMakie only).
gap
Defaults to 0.2
The final width of the bars is calculated as w * (1 - gap)
where w
is the width of each bar as determined with the width
attribute.
highclip
Defaults to automatic
The color for any value above the colorrange.
inspectable
Defaults to @inherit inspectable
Sets whether this plot should be seen by DataInspector
. The default depends on the theme of the parent scene.
inspector_clear
Defaults to automatic
Sets a callback function (inspector, plot) -> ...
for cleaning up custom indicators in DataInspector.
inspector_hover
Defaults to automatic
Sets a callback function (inspector, plot, index) -> ...
which replaces the default show_data
methods.
inspector_label
Defaults to automatic
Sets a callback function (plot, index, position) -> string
which replaces the default label generated by DataInspector.
label_align
Defaults to automatic
No docs available.
label_color
Defaults to @inherit textcolor
No docs available.
label_font
Defaults to @inherit font
The font of the bar labels.
label_formatter
Defaults to bar_label_formatter
No docs available.
label_offset
Defaults to 5
The distance of the labels from the bar ends in screen units. Does not apply when label_position = :center
.
label_position
Defaults to :end
The position of each bar's label relative to the bar. Possible values are :end
or :center
.
label_rotation
Defaults to 0π
No docs available.
label_size
Defaults to @inherit fontsize
The font size of the bar labels.
lowclip
Defaults to automatic
The color for any value below the colorrange.
model
Defaults to automatic
Sets a model matrix for the plot. This overrides adjustments made with translate!
, rotate!
and scale!
.
n_dodge
Defaults to automatic
No docs available.
nan_color
Defaults to :transparent
The color for NaN values.
offset
Defaults to 0.0
No docs available.
overdraw
Defaults to false
Controls if the plot will draw over other plots. This specifically means ignoring depth checks in GL backends
space
Defaults to :data
Sets the transformation space for box encompassing the plot. See Makie.spaces()
for possible inputs.
ssao
Defaults to false
Adjusts whether the plot is rendered with ssao (screen space ambient occlusion). Note that this only makes sense in 3D plots and is only applicable with fxaa = true
.
stack
Defaults to automatic
No docs available.
strokecolor
Defaults to @inherit patchstrokecolor
No docs available.
strokewidth
Defaults to @inherit patchstrokewidth
No docs available.
transformation
Defaults to :automatic
No docs available.
transparency
Defaults to false
Adjusts how the plot deals with transparency. In GLMakie transparency = true
results in using Order Independent Transparency.
visible
Defaults to true
Controls whether the plot will be rendered or not.
width
Defaults to automatic
The gapless width of the bars. If automatic
, the width w
is calculated as minimum(diff(sort(unique(positions)))
. The actual width of the bars is calculated as w * (1 - gap)
.