waterfall
Makie.waterfall Function
waterfall(x, y; kwargs...)
Plots a waterfall chart to visualize individual positive and negative components that add up to a net result as a barplot with stacked bars next to each other.
Plot type
The plot type alias for the waterfall
function is Waterfall
.
Examples
using CairoMakie
y = [6, 4, 2, -8, 3, 5, 1, -2, -3, 7]
waterfall(y)
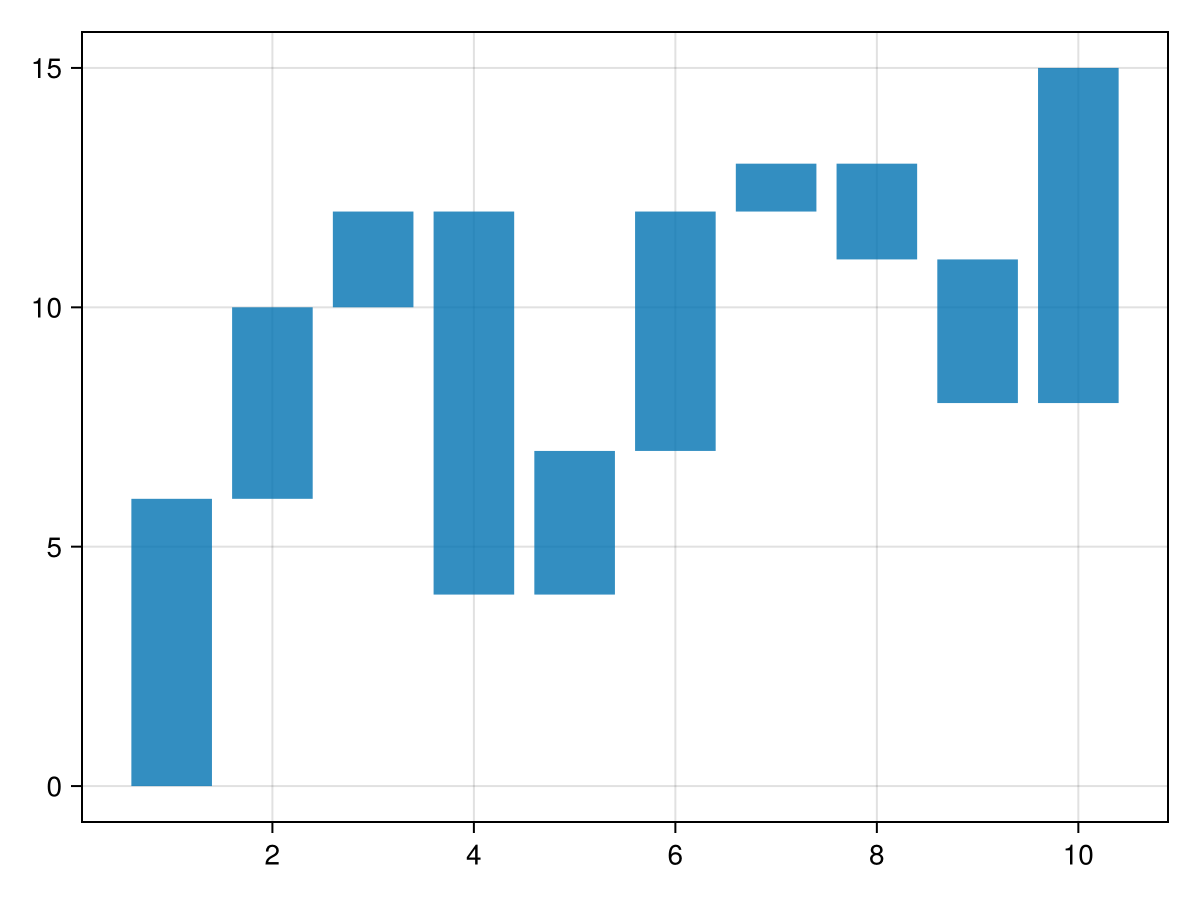
The direction of the bars might be easier to parse with some visual support.
using CairoMakie
y = [6, 4, 2, -8, 3, 5, 1, -2, -3, 7]
waterfall(y, show_direction=true)
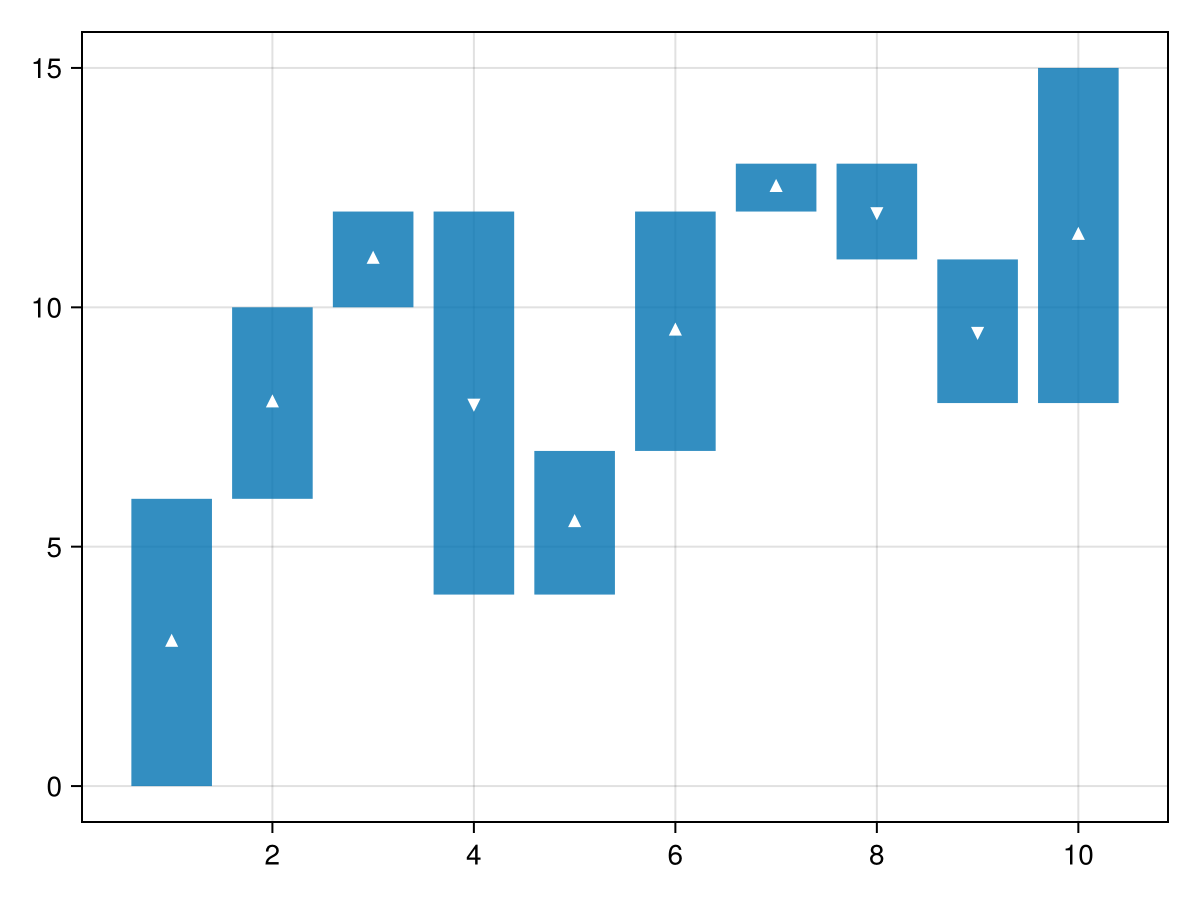
You can customize the markers that indicate the bar directions.
using CairoMakie
y = [6, 4, 2, -8, 3, 5, 1, -2, -3, 7]
waterfall(y, show_direction=true, marker_pos=:cross, marker_neg=:hline, direction_color=:gold)
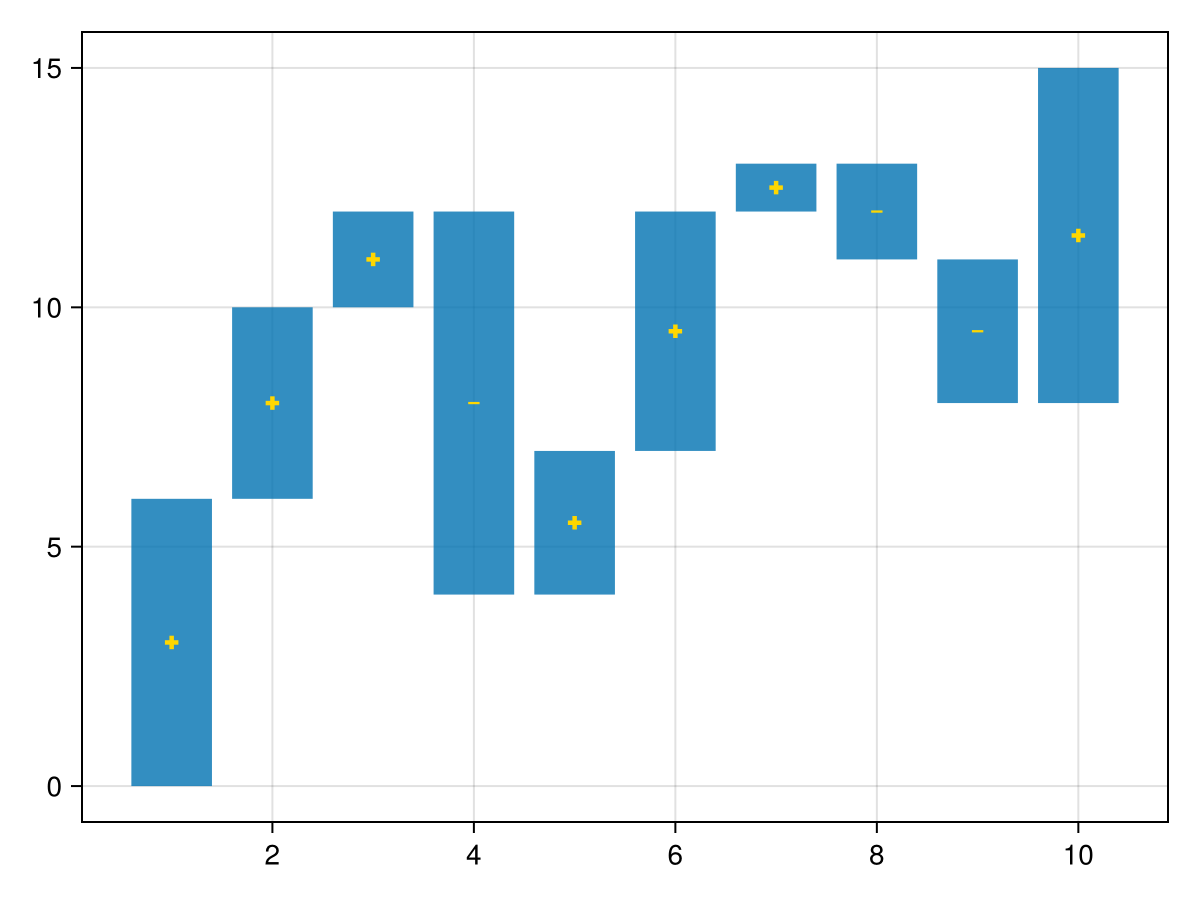
If the dodge
attribute is provided, bars are stacked by dodge
.
using CairoMakie
colors = Makie.wong_colors()
x = repeat(1:2, inner=5)
y = [6, 4, 2, -8, 3, 5, 1, -2, -3, 7]
group = repeat(1:5, outer=2)
waterfall(x, y, dodge=group, color=colors[group])
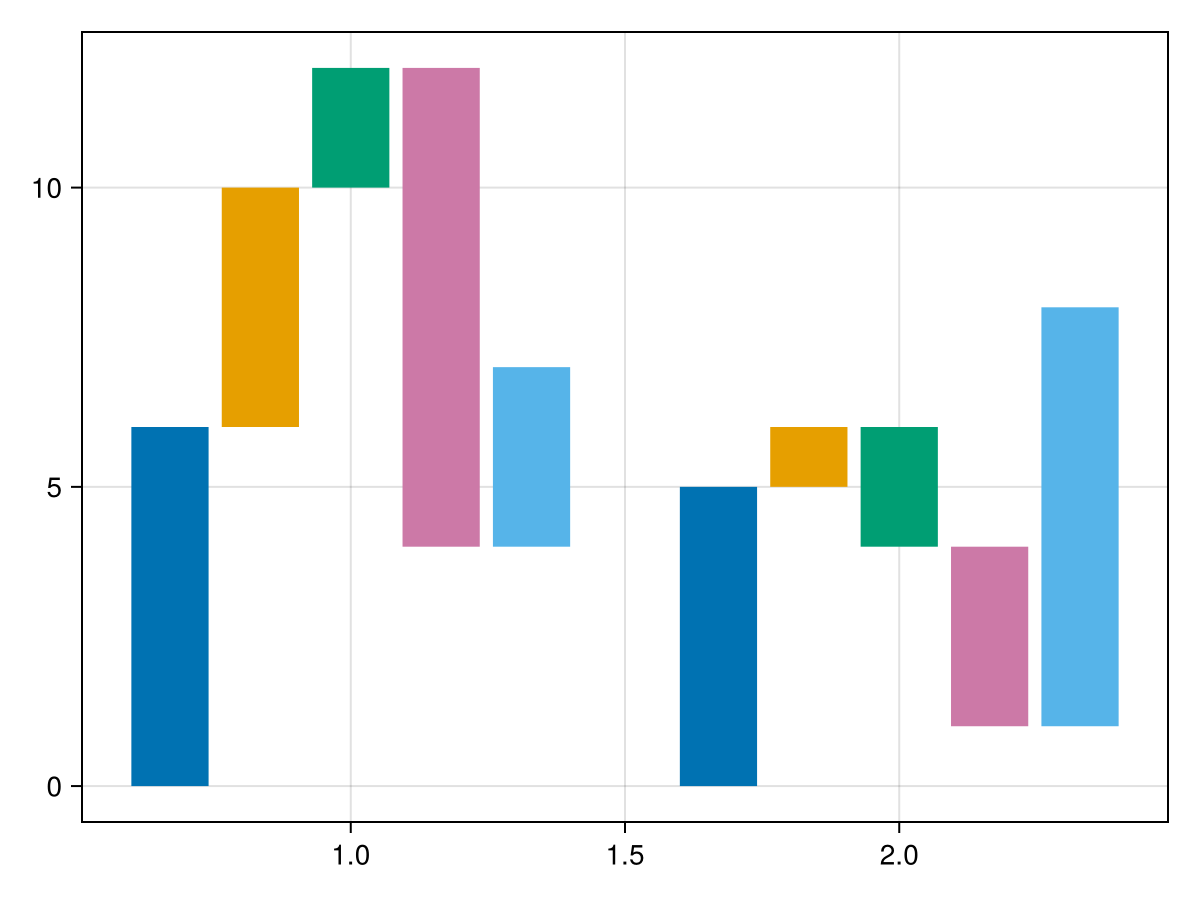
It can be easier to compare final results of different groups if they are shown in the background.
using CairoMakie
colors = Makie.wong_colors()
x = repeat(1:2, inner=5)
y = [6, 4, 2, -8, 3, 5, 1, -2, -3, 7]
group = repeat(1:5, outer=2)
waterfall(x, y, dodge=group, color=colors[group], show_direction=true, show_final=true)
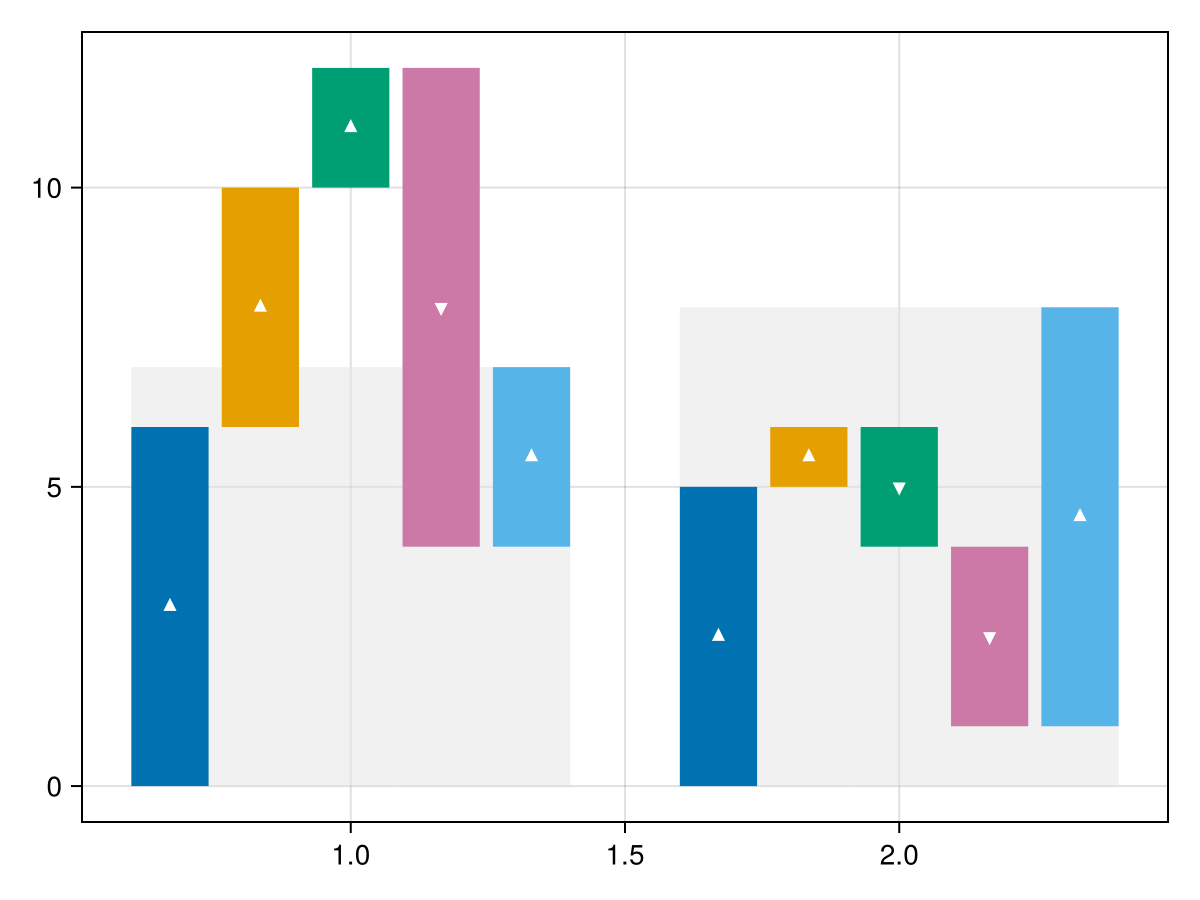
The color of the final bars in the background can be modified.
using CairoMakie
colors = Makie.wong_colors()
x = repeat(1:2, inner=5)
y = [6, 4, 2, -8, 3, 5, 1, -2, -3, 7]
group = repeat(1:5, outer=2)
waterfall(x, y, dodge=group, color=colors[group], show_final=true, final_color=(colors[6], 1//3))
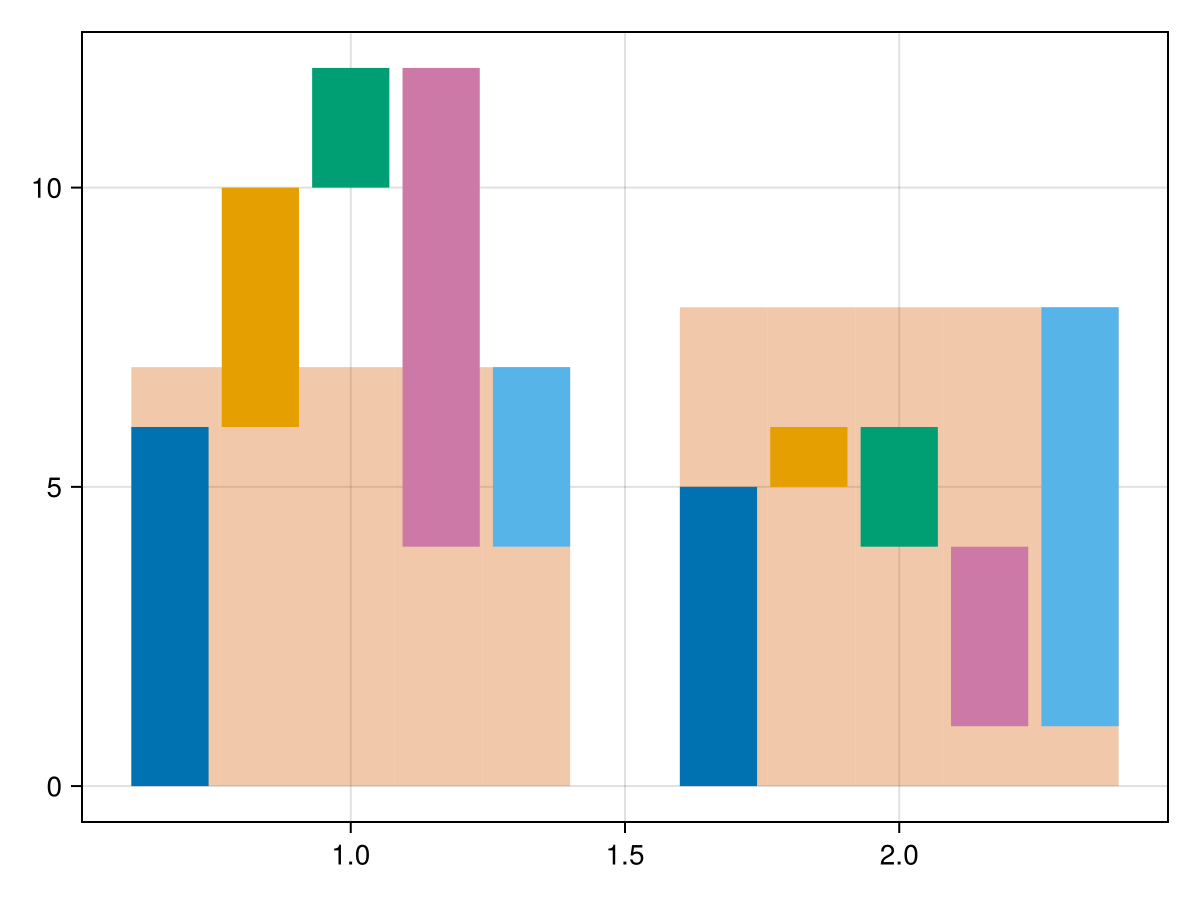
You can also specify to stack grouped waterfall plots by x
.
using CairoMakie
colors = Makie.wong_colors()
x = repeat(1:5, outer=2)
y = [6, 4, 2, -8, 3, 5, 1, -2, -3, 7]
group = repeat(1:2, inner=5)
waterfall(x, y, dodge=group, color=colors[group], show_direction=true, stack=:x)
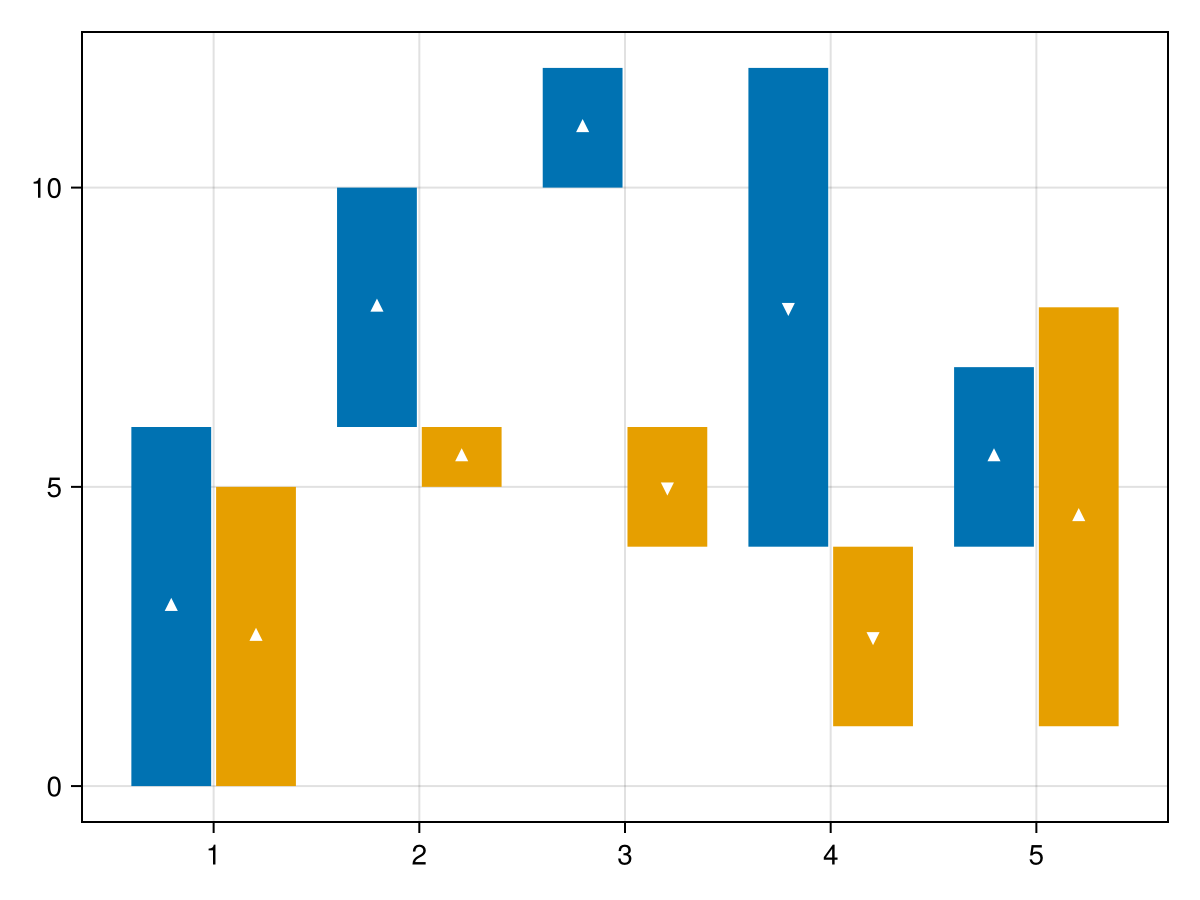
Attributes
color
Defaults to @inherit patchcolor
No docs available.
cycle
Defaults to [:color => :patchcolor]
No docs available.
direction_color
Defaults to @inherit backgroundcolor
No docs available.
dodge
Defaults to automatic
No docs available.
dodge_gap
Defaults to 0.03
No docs available.
final_color
Defaults to plot_color(:grey90, 0.5)
No docs available.
final_dodge_gap
Defaults to 0
No docs available.
final_gap
Defaults to automatic
No docs available.
gap
Defaults to 0.2
No docs available.
marker_neg
Defaults to :dtriangle
No docs available.
marker_pos
Defaults to :utriangle
No docs available.
n_dodge
Defaults to automatic
No docs available.
show_direction
Defaults to false
No docs available.
show_final
Defaults to false
No docs available.
stack
Defaults to automatic
No docs available.
width
Defaults to automatic
No docs available.