linesegments
MakieCore.linesegments Function
linesegments(positions)
linesegments(vector_of_2tuples_of_points)
linesegments(x, y)
linesegments(x, y, z)
Plots a line for each pair of points in (x, y, z)
, (x, y)
, or positions
.
Plot type
The plot type alias for the linesegments
function is LineSegments
.
Examples
using CairoMakie
f = Figure()
Axis(f[1, 1])
xs = 1:0.2:10
ys = sin.(xs)
linesegments!(xs, ys)
linesegments!(xs, ys .- 1, linewidth = 5)
linesegments!(xs, ys .- 2, linewidth = 5, color = LinRange(1, 5, length(xs)))
f
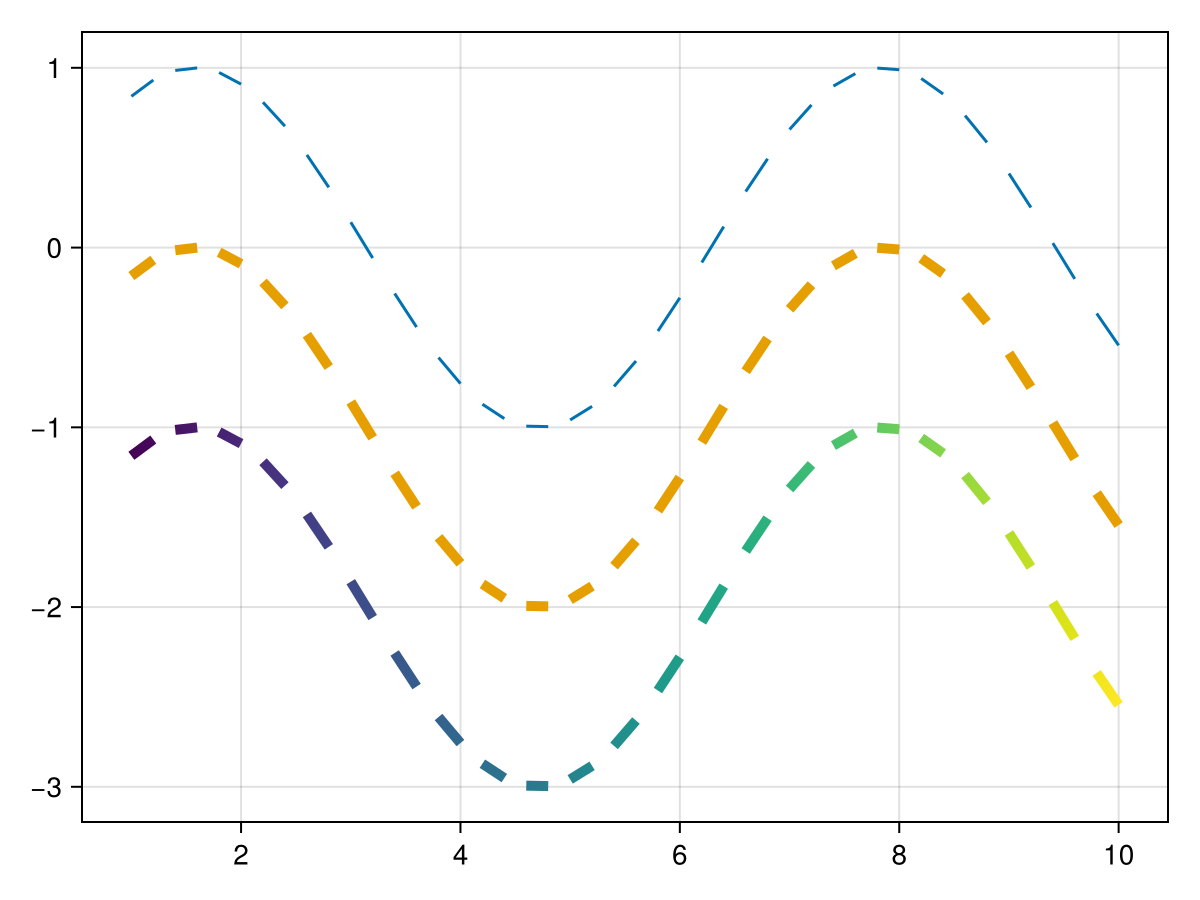
Dealing with outline artifacts in GLMakie
In GLMakie 3D line plots can generate outline artifacts depending on the order line segments are rendered in. Currently there are a few ways to mitigate this problem, but they all come at a cost:
fxaa = true
will disable the native anti-aliasing of line segments and use fxaa instead. This results in less detailed lines.transparency = true
will disable depth testing to a degree, resulting in all lines being rendered without artifacts. However with this lines will always have some level of transparency.overdraw = true
will disable depth testing entirely (read and write) for the plot, removing artifacts. This will however change the z-order of line segments and allow plots rendered later to show up on top of the linesegments plot.
using GLMakie
ps = rand(Point3f, 500)
cs = rand(500)
f = Figure(size = (600, 650))
Label(f[1, 1], "base", tellwidth = false)
linesegments(f[2, 1], ps, color = cs, fxaa = false)
Label(f[1, 2], "fxaa = true", tellwidth = false)
linesegments(f[2, 2], ps, color = cs, fxaa = true)
Label(f[3, 1], "transparency = true", tellwidth = false)
linesegments(f[4, 1], ps, color = cs, transparency = true)
Label(f[3, 2], "overdraw = true", tellwidth = false)
linesegments(f[4, 2], ps, color = cs, overdraw = true)
f
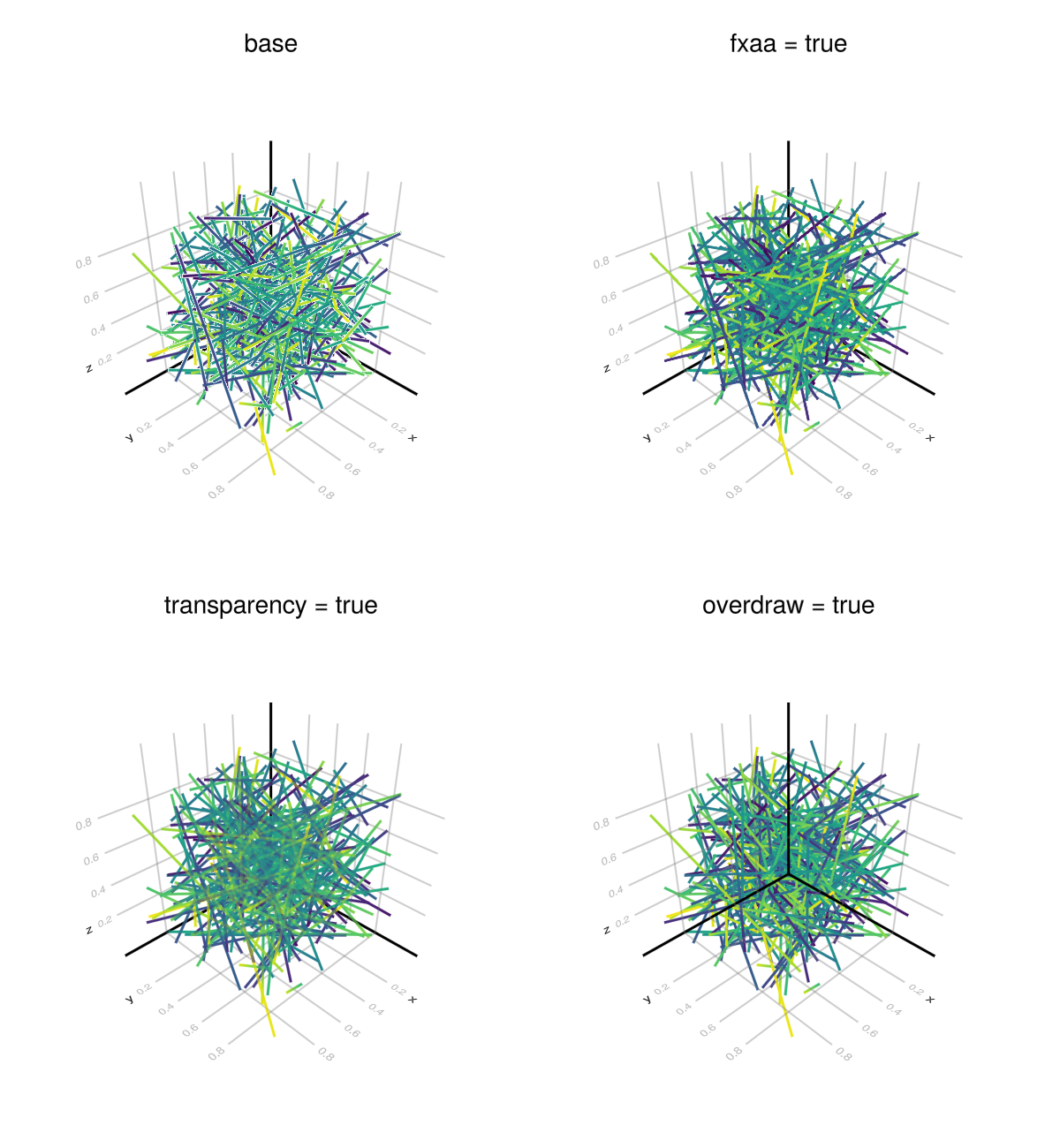
Attributes
alpha
Defaults to 1.0
The alpha value of the colormap or color attribute. Multiple alphas like in plot(alpha=0.2, color=(:red, 0.5)
, will get multiplied.
clip_planes
Defaults to automatic
Clip planes offer a way to do clipping in 3D space. You can set a Vector of up to 8 Plane3f
planes here, behind which plots will be clipped (i.e. become invisible). By default clip planes are inherited from the parent plot or scene. You can remove parent clip_planes
by passing Plane3f[]
.
color
Defaults to @inherit linecolor
The color of the line.
colormap
Defaults to @inherit colormap :viridis
Sets the colormap that is sampled for numeric color
s. PlotUtils.cgrad(...)
, Makie.Reverse(any_colormap)
can be used as well, or any symbol from ColorBrewer or PlotUtils. To see all available color gradients, you can call Makie.available_gradients()
.
colorrange
Defaults to automatic
The values representing the start and end points of colormap
.
colorscale
Defaults to identity
The color transform function. Can be any function, but only works well together with Colorbar
for identity
, log
, log2
, log10
, sqrt
, logit
, Makie.pseudolog10
and Makie.Symlog10
.
cycle
Defaults to [:color]
Sets which attributes to cycle when creating multiple plots.
depth_shift
Defaults to 0.0
adjusts the depth value of a plot after all other transformations, i.e. in clip space, where 0 <= depth <= 1
. This only applies to GLMakie and WGLMakie and can be used to adjust render order (like a tunable overdraw).
fxaa
Defaults to false
adjusts whether the plot is rendered with fxaa (anti-aliasing, GLMakie only).
highclip
Defaults to automatic
The color for any value above the colorrange.
inspectable
Defaults to true
sets whether this plot should be seen by DataInspector
.
inspector_clear
Defaults to automatic
Sets a callback function (inspector, plot) -> ...
for cleaning up custom indicators in DataInspector.
inspector_hover
Defaults to automatic
Sets a callback function (inspector, plot, index) -> ...
which replaces the default show_data
methods.
inspector_label
Defaults to automatic
Sets a callback function (plot, index, position) -> string
which replaces the default label generated by DataInspector.
linecap
Defaults to @inherit linecap
Sets the type of linecap used, i.e. :butt (flat with no extrusion), :square (flat with 1 linewidth extrusion) or :round.
linestyle
Defaults to nothing
Sets the dash pattern of the line. Options are :solid
(equivalent to nothing
), :dot
, :dash
, :dashdot
and :dashdotdot
. These can also be given in a tuple with a gap style modifier, either :normal
, :dense
or :loose
. For example, (:dot, :loose)
or (:dashdot, :dense)
.
For custom patterns have a look at Makie.Linestyle
.
linewidth
Defaults to @inherit linewidth
Sets the width of the line in pixel units
lowclip
Defaults to automatic
The color for any value below the colorrange.
model
Defaults to automatic
Sets a model matrix for the plot. This overrides adjustments made with translate!
, rotate!
and scale!
.
nan_color
Defaults to :transparent
The color for NaN values.
overdraw
Defaults to false
Controls if the plot will draw over other plots. This specifically means ignoring depth checks in GL backends
space
Defaults to :data
sets the transformation space for box encompassing the plot. See Makie.spaces()
for possible inputs.
ssao
Defaults to false
Adjusts whether the plot is rendered with ssao (screen space ambient occlusion). Note that this only makes sense in 3D plots and is only applicable with fxaa = true
.
transformation
Defaults to automatic
No docs available.
transparency
Defaults to false
Adjusts how the plot deals with transparency. In GLMakie transparency = true
results in using Order Independent Transparency.
visible
Defaults to true
Controls whether the plot will be rendered or not.