Label
A Label is text within a rectangular boundingbox. The halign
and valign
attributes always refer to unrotated horizontal and vertical. This is different from text
, where alignment is relative to text flow direction.
A Label's size is known, so if tellwidth
and tellheight
are set to true
(the default values) a GridLayout with Auto
column or row sizes can shrink to fit.
using CairoMakie
fig = Figure()
fig[1:2, 1:3] = [Axis(fig) for _ in 1:6]
supertitle = Label(fig[0, :], "Six plots", fontsize = 30)
sideinfo = Label(fig[1:2, 0], "This text is vertical", rotation = pi/2)
fig
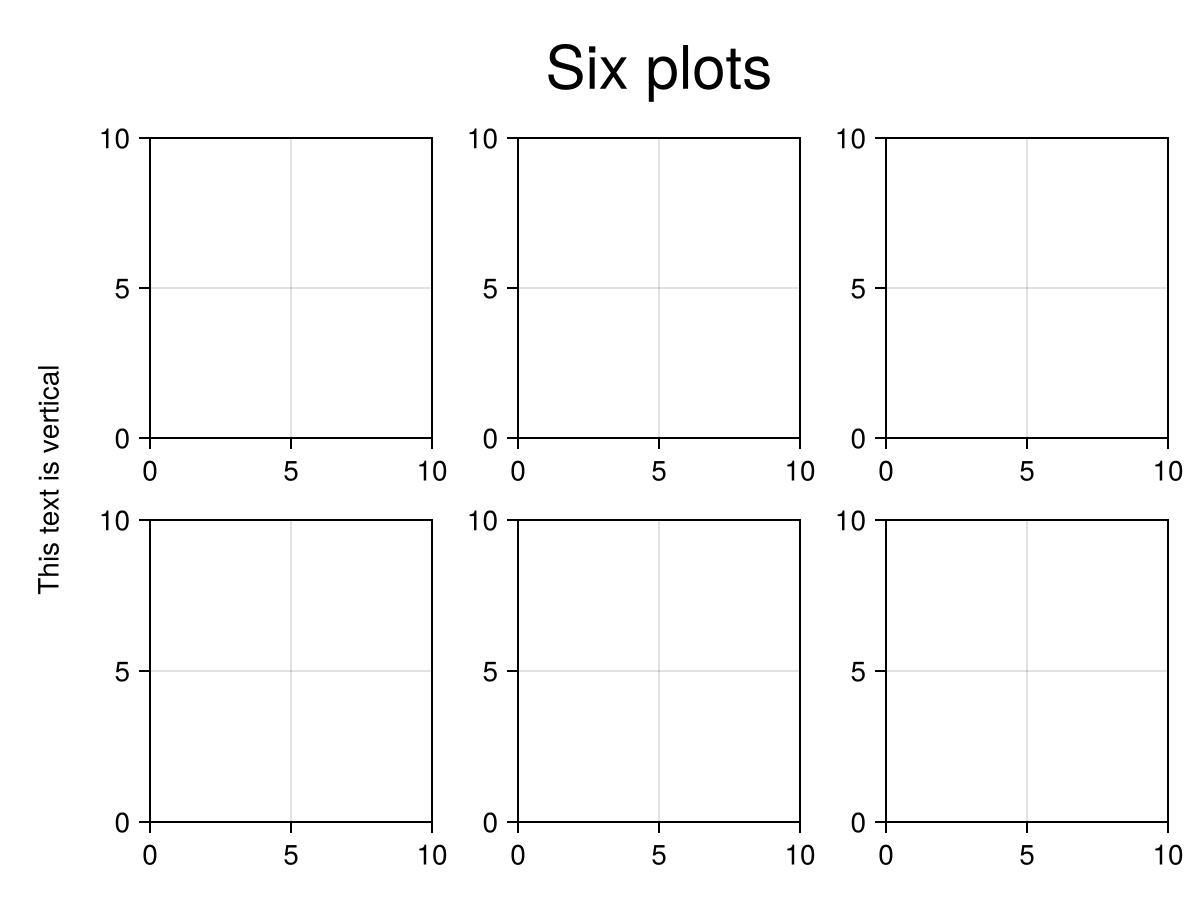
Justification and lineheight of a label can be controlled just like with normal text.
using CairoMakie
f = Figure()
Label(f[1, 1],
"Multiline label\nwith\njustification = :left\nand\nlineheight = 0.9",
justification = :left,
lineheight = 0.9
)
Label(f[1, 2],
"Multiline label\nwith\njustification = :center\nand\nlineheight = 1.1",
justification = :center,
lineheight = 1.1,
color = :dodgerblue,
)
Label(f[1, 3],
"Multiline label\nwith\njustification = :right\nand\nlineheight = 1.3",
justification = :right,
lineheight = 1.3,
color = :firebrick
)
f
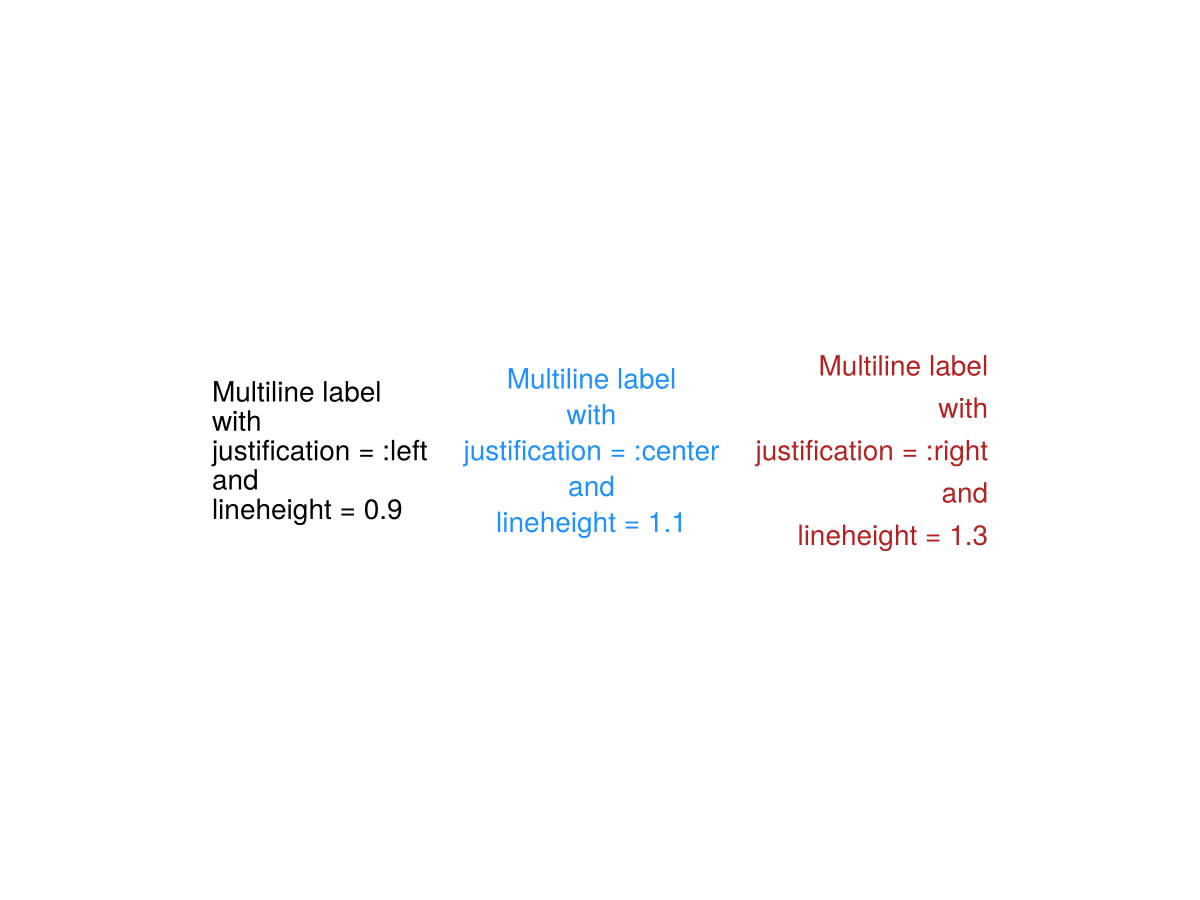
Attributes
alignmode
Defaults to Inside()
The align mode of the text in its parent GridLayout.
color
Defaults to @inherit :textcolor :black
The color of the text.
font
Defaults to :regular
The font family of the text.
fontsize
Defaults to @inherit :fontsize 16.0f0
The font size of the text.
halign
Defaults to :center
The horizontal alignment of the text in its suggested boundingbox
height
Defaults to Auto()
The height setting of the text.
justification
Defaults to :center
The justification of the text (:left, :right, :center).
lineheight
Defaults to 1.0
The lineheight multiplier for the text.
padding
Defaults to (0.0f0, 0.0f0, 0.0f0, 0.0f0)
The extra space added to the sides of the text boundingbox.
rotation
Defaults to 0.0
The counterclockwise rotation of the text in radians.
tellheight
Defaults to true
Controls if the parent layout can adjust to this element's height
tellwidth
Defaults to true
Controls if the parent layout can adjust to this element's width
text
Defaults to "Text"
The displayed text string.
valign
Defaults to :center
The vertical alignment of the text in its suggested boundingbox
visible
Defaults to true
Controls if the text is visible.
width
Defaults to Auto()
The width setting of the text.
word_wrap
Defaults to false
Enable word wrapping to the suggested width of the Label.