streamplot
Makie.streamplot Function
streamplot(f::function, xinterval, yinterval; color = norm, kwargs...)
f must either accept f(::Point)
or f(x::Number, y::Number)
. f must return a Point2.
Example:
v(x::Point2{T}) where T = Point2f(x[2], 4*x[1])
streamplot(v, -2..2, -2..2)
Implementation
See the function Makie.streamplot_impl
for implementation details.
Plot type
The plot type alias for the streamplot
function is StreamPlot
.
Examples
using CairoMakie
struct FitzhughNagumo{T}
ϵ::T
s::T
γ::T
β::T
end
P = FitzhughNagumo(0.1, 0.0, 1.5, 0.8)
f(x, P::FitzhughNagumo) = Point2f(
(x[1]-x[2]-x[1]^3+P.s)/P.ϵ,
P.γ*x[1]-x[2] + P.β
)
f(x) = f(x, P)
fig, ax, pl = streamplot(f, -1.5..1.5, -1.5..1.5, colormap = :magma)
# you can also pass a function to `color`, to either return a number or color value
streamplot(fig[1,2], f, -1.5 .. 1.5, -1.5 .. 1.5, color=(p)-> RGBAf(p..., 0.0, 1))
fig
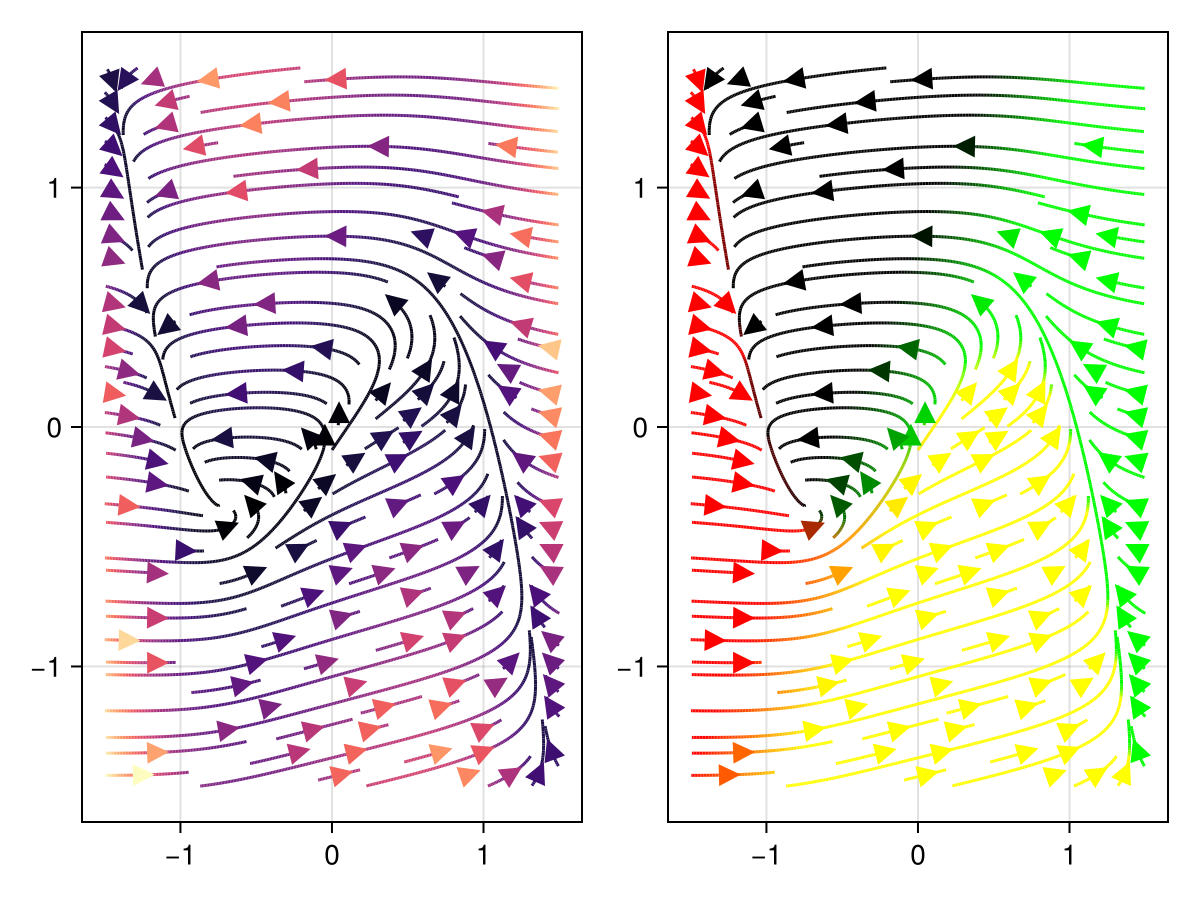
Attributes
alpha
Defaults to 1.0
The alpha value of the colormap or color attribute. Multiple alphas like in plot(alpha=0.2, color=(:red, 0.5)
, will get multiplied.
arrow_head
Defaults to automatic
No docs available.
arrow_size
Defaults to automatic
No docs available.
clip_planes
Defaults to automatic
Clip planes offer a way to do clipping in 3D space. You can set a Vector of up to 8 Plane3f
planes here, behind which plots will be clipped (i.e. become invisible). By default clip planes are inherited from the parent plot or scene. You can remove parent clip_planes
by passing Plane3f[]
.
color
Defaults to norm
One can choose the color of the lines by passing a function color_func(dx::Point)
to the color
attribute. This can be set to any function or composition of functions. The dx
which is passed to color_func
is the output of f
at the point being colored.
colormap
Defaults to @inherit colormap :viridis
Sets the colormap that is sampled for numeric color
s. PlotUtils.cgrad(...)
, Makie.Reverse(any_colormap)
can be used as well, or any symbol from ColorBrewer or PlotUtils. To see all available color gradients, you can call Makie.available_gradients()
.
colorrange
Defaults to automatic
The values representing the start and end points of colormap
.
colorscale
Defaults to identity
The color transform function. Can be any function, but only works well together with Colorbar
for identity
, log
, log2
, log10
, sqrt
, logit
, Makie.pseudolog10
and Makie.Symlog10
.
density
Defaults to 1.0
No docs available.
depth_shift
Defaults to 0.0
adjusts the depth value of a plot after all other transformations, i.e. in clip space, where 0 <= depth <= 1
. This only applies to GLMakie and WGLMakie and can be used to adjust render order (like a tunable overdraw).
fxaa
Defaults to true
adjusts whether the plot is rendered with fxaa (anti-aliasing, GLMakie only).
gridsize
Defaults to (32, 32, 32)
No docs available.
highclip
Defaults to automatic
The color for any value above the colorrange.
inspectable
Defaults to true
sets whether this plot should be seen by DataInspector
.
inspector_clear
Defaults to automatic
Sets a callback function (inspector, plot) -> ...
for cleaning up custom indicators in DataInspector.
inspector_hover
Defaults to automatic
Sets a callback function (inspector, plot, index) -> ...
which replaces the default show_data
methods.
inspector_label
Defaults to automatic
Sets a callback function (plot, index, position) -> string
which replaces the default label generated by DataInspector.
joinstyle
Defaults to @inherit joinstyle
No docs available.
linecap
Defaults to @inherit linecap
No docs available.
linestyle
Defaults to nothing
No docs available.
linewidth
Defaults to @inherit linewidth
No docs available.
lowclip
Defaults to automatic
The color for any value below the colorrange.
maxsteps
Defaults to 500
No docs available.
miter_limit
Defaults to @inherit miter_limit
No docs available.
model
Defaults to automatic
Sets a model matrix for the plot. This overrides adjustments made with translate!
, rotate!
and scale!
.
nan_color
Defaults to :transparent
The color for NaN values.
overdraw
Defaults to false
Controls if the plot will draw over other plots. This specifically means ignoring depth checks in GL backends
quality
Defaults to 16
No docs available.
space
Defaults to :data
sets the transformation space for box encompassing the plot. See Makie.spaces()
for possible inputs.
ssao
Defaults to false
Adjusts whether the plot is rendered with ssao (screen space ambient occlusion). Note that this only makes sense in 3D plots and is only applicable with fxaa = true
.
stepsize
Defaults to 0.01
No docs available.
transformation
Defaults to automatic
No docs available.
transparency
Defaults to false
Adjusts how the plot deals with transparency. In GLMakie transparency = true
results in using Order Independent Transparency.
visible
Defaults to true
Controls whether the plot will be rendered or not.