arc
Makie.arc Function
arc(origin, radius, start_angle, stop_angle; kwargs...)
This function plots a circular arc, centered at origin
with radius radius
, from start_angle
to stop_angle
. origin
must be a coordinate in 2 dimensions (i.e., a Point2
); the rest of the arguments must be <: Number
.
Examples:
arc(Point2f(0), 1, 0.0, π)
arc(Point2f(1, 2), 0.3, π, -π)
Plot type
The plot type alias for the arc
function is Arc
.
Examples
using CairoMakie
arc(Point2f(0), 1, -π, π)
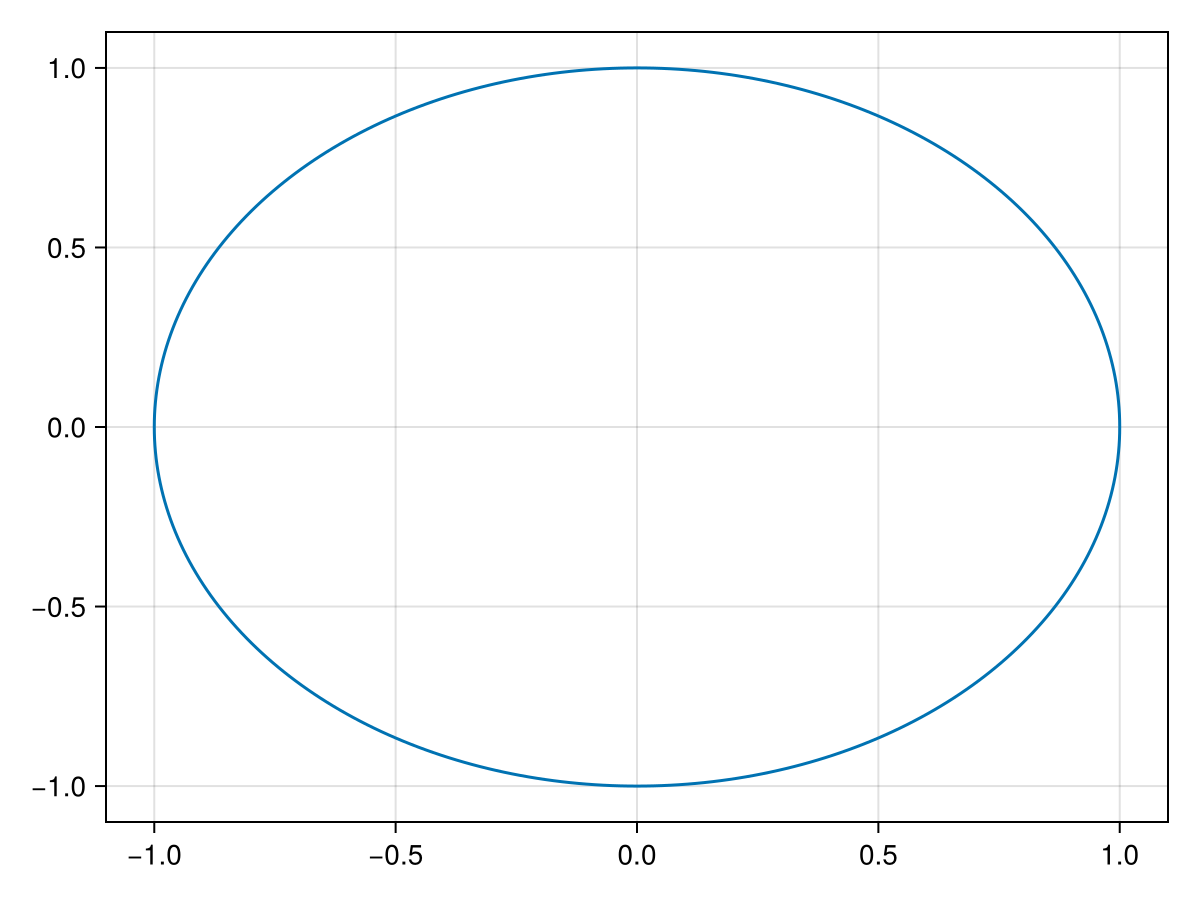
using CairoMakie
f = Figure()
Axis(f[1, 1])
for i in 1:10
arc!(Point2f(0, i), i, -π, π)
end
f
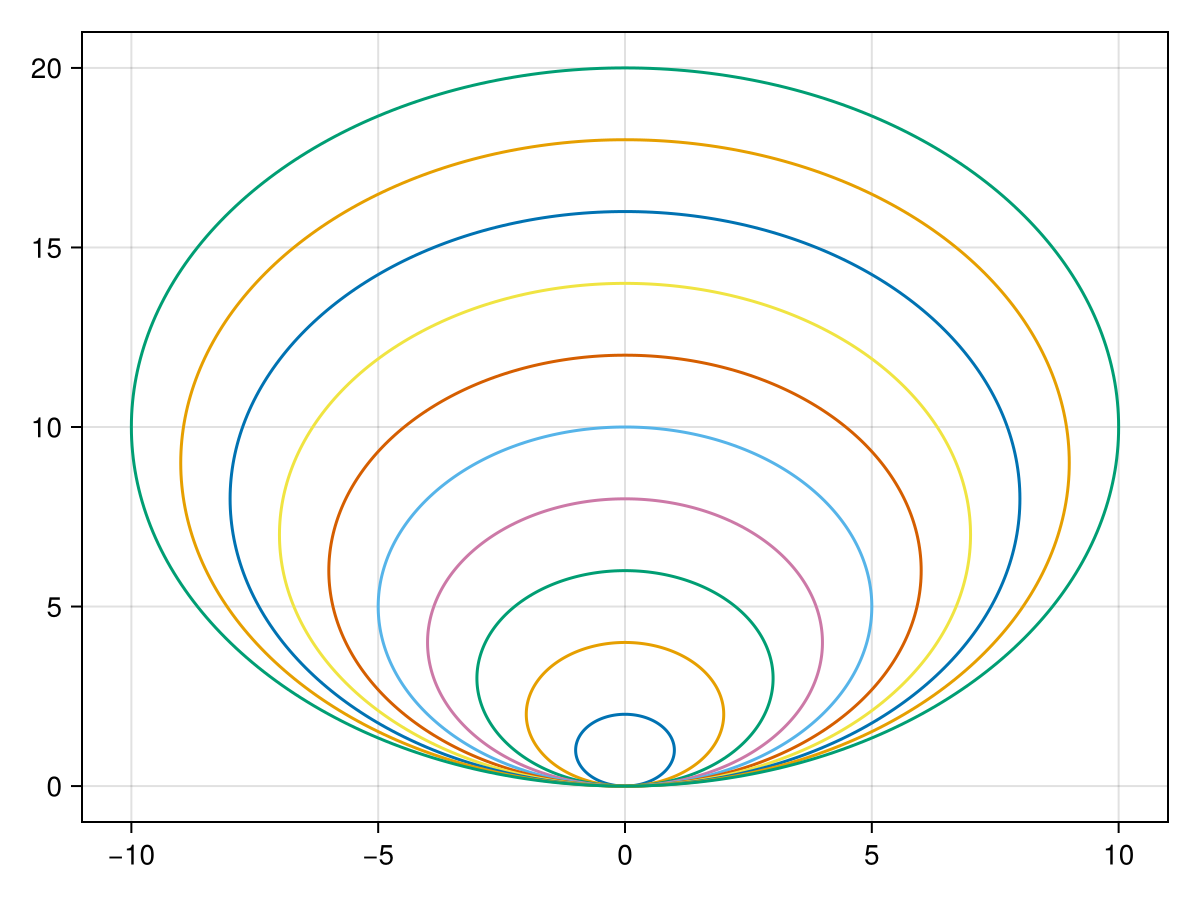
using CairoMakie
f = Figure()
Axis(f[1, 1])
for i in 1:4
radius = 1/(i*2)
left = 1/(i*2)
right = (i*2-1)/(i*2)
arc!(Point2f(left, 0), radius, 0, π)
arc!(Point2f(right, 0), radius, 0, π)
end
for i in 3:4
radius = 1/(i*(i-1)*2)
left = (1/i) + 1/(i*(i-1)*2)
right = ((i-1)/i) - 1/(i*(i-1)*2)
arc!(Point2f(left, 0), radius, 0, π)
arc!(Point2f(right, 0), radius, 0, π)
end
f
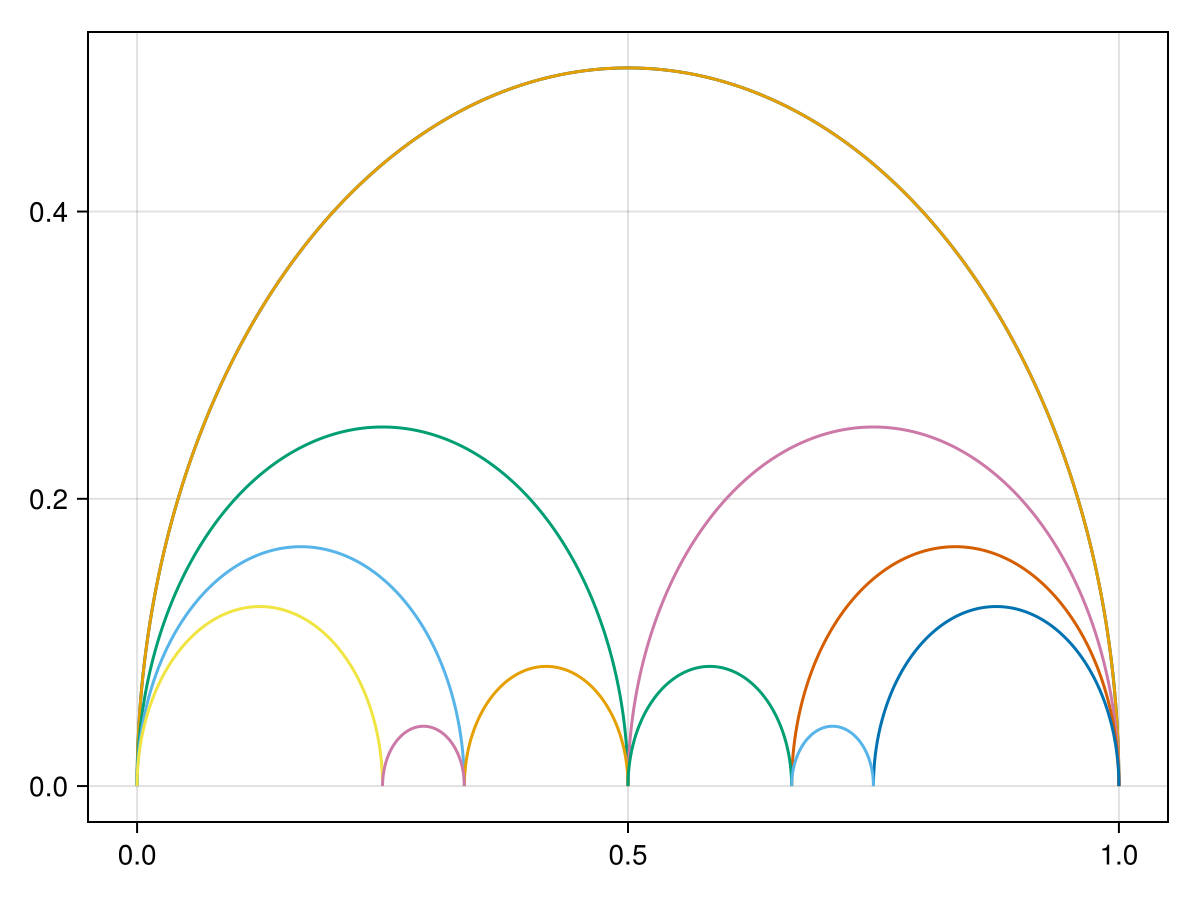
Attributes
alpha
Defaults to 1.0
The alpha value of the colormap or color attribute. Multiple alphas like in plot(alpha=0.2, color=(:red, 0.5)
, will get multiplied.
clip_planes
Defaults to @inherit clip_planes automatic
Clip planes offer a way to do clipping in 3D space. You can set a Vector of up to 8 Plane3f
planes here, behind which plots will be clipped (i.e. become invisible). By default clip planes are inherited from the parent plot or scene. You can remove parent clip_planes
by passing Plane3f[]
.
color
Defaults to @inherit linecolor
The color of the line.
colormap
Defaults to @inherit colormap :viridis
Sets the colormap that is sampled for numeric color
s. PlotUtils.cgrad(...)
, Makie.Reverse(any_colormap)
can be used as well, or any symbol from ColorBrewer or PlotUtils. To see all available color gradients, you can call Makie.available_gradients()
.
colorrange
Defaults to automatic
The values representing the start and end points of colormap
.
colorscale
Defaults to identity
The color transform function. Can be any function, but only works well together with Colorbar
for identity
, log
, log2
, log10
, sqrt
, logit
, Makie.pseudolog10
and Makie.Symlog10
.
cycle
Defaults to [:color]
Sets which attributes to cycle when creating multiple plots.
depth_shift
Defaults to 0.0
Adjusts the depth value of a plot after all other transformations, i.e. in clip space, where -1 <= depth <= 1
. This only applies to GLMakie and WGLMakie and can be used to adjust render order (like a tunable overdraw).
fxaa
Defaults to false
Adjusts whether the plot is rendered with fxaa (anti-aliasing, GLMakie only).
highclip
Defaults to automatic
The color for any value above the colorrange.
inspectable
Defaults to @inherit inspectable
Sets whether this plot should be seen by DataInspector
. The default depends on the theme of the parent scene.
inspector_clear
Defaults to automatic
Sets a callback function (inspector, plot) -> ...
for cleaning up custom indicators in DataInspector.
inspector_hover
Defaults to automatic
Sets a callback function (inspector, plot, index) -> ...
which replaces the default show_data
methods.
inspector_label
Defaults to automatic
Sets a callback function (plot, index, position) -> string
which replaces the default label generated by DataInspector.
joinstyle
Defaults to @inherit joinstyle
Controls the rendering at corners. Options are :miter
for sharp corners, :bevel
for "cut off" corners, and :round
for rounded corners. If the corner angle is below miter_limit
, :miter
is equivalent to :bevel
to avoid long spikes.
linecap
Defaults to @inherit linecap
Sets the type of line cap used. Options are :butt
(flat without extrusion), :square
(flat with half a linewidth extrusion) or :round
.
linestyle
Defaults to nothing
Sets the dash pattern of the line. Options are :solid
(equivalent to nothing
), :dot
, :dash
, :dashdot
and :dashdotdot
. These can also be given in a tuple with a gap style modifier, either :normal
, :dense
or :loose
. For example, (:dot, :loose)
or (:dashdot, :dense)
.
For custom patterns have a look at Makie.Linestyle
.
linewidth
Defaults to @inherit linewidth
Sets the width of the line in screen units
lowclip
Defaults to automatic
The color for any value below the colorrange.
miter_limit
Defaults to @inherit miter_limit
Sets the minimum inner join angle below which miter joins truncate. See also Makie.miter_distance_to_angle
.
model
Defaults to automatic
Sets a model matrix for the plot. This overrides adjustments made with translate!
, rotate!
and scale!
.
nan_color
Defaults to :transparent
The color for NaN values.
overdraw
Defaults to false
Controls if the plot will draw over other plots. This specifically means ignoring depth checks in GL backends
resolution
Defaults to 361
The number of line points approximating the arc.
space
Defaults to :data
Sets the transformation space for box encompassing the plot. See Makie.spaces()
for possible inputs.
ssao
Defaults to false
Adjusts whether the plot is rendered with ssao (screen space ambient occlusion). Note that this only makes sense in 3D plots and is only applicable with fxaa = true
.
transformation
Defaults to :automatic
No docs available.
transparency
Defaults to false
Adjusts how the plot deals with transparency. In GLMakie transparency = true
results in using Order Independent Transparency.
visible
Defaults to true
Controls whether the plot will be rendered or not.